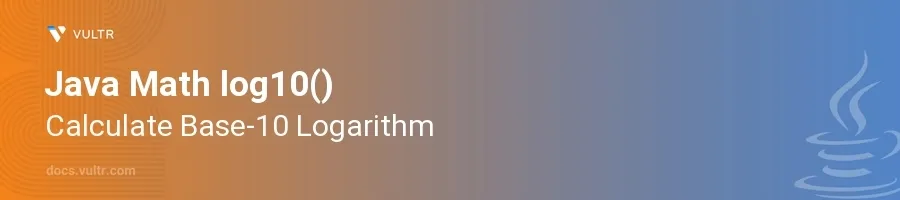
Introduction
The log10()
method in Java is part of the Math class and is used to calculate the logarithm of a number with base 10. This method is particularly useful in scientific calculations where logarithmic scales are necessary, such as in sound intensity levels, pH calculations in chemistry, and data scaling in statistics.
In this article, you will learn how to use the log10()
method to compute base-10 logarithms of various numbers. Explore how to handle different cases, including positive values, zero, and negative numbers, and learn how these scenarios affect the outcome of your calculations.
Understanding Math log10()
Calculate the Base-10 Log of a Positive Number
Start by defining a positive numeric value.
Use
Math.log10()
to calculate the logarithm of the number.javadouble result = Math.log10(100); System.out.println("Log10 of 100 is: " + result);
This code calculates the base-10 logarithm of
100
. The output will be2.0
, since (10^2 = 100).
Handling Zero and Negative Inputs
Understand the behavior of
log10()
with zero and negative inputs.- Zero: Mathematically, the log of zero is undefined in the real number system. In Java, passing zero to
log10()
results in negative infinity. - Negative numbers: Like zero, the log of a negative number is also not defined in the real number system. Java returns NaN, which stands for "Not a Number."
- Zero: Mathematically, the log of zero is undefined in the real number system. In Java, passing zero to
Demonstrate these outcomes with appropriate examples.
javadouble logZero = Math.log10(0); double logNegative = Math.log10(-50); System.out.println("Log10 of 0 is: " + logZero); System.out.println("Log10 of -50 is: " + logNegative);
This example outputs
Log10 of 0 is: -Infinity
andLog10 of -50 is: NaN
. This showcases how Java handles these situations.
Special Cases and Practical Applications
Calculate Logarithms with Non-Integer Values
Recognize that
log10()
can handle non-integer and positive decimal values efficiently.Calculate the base-10 log of a non-integer.
javadouble result = Math.log10(2.71828); System.out.println("Log10 of 2.71828 is: " + result);
This output, approximately
0.4343
, demonstrates the logarithm of a non-integer number.
Practical Usage in Scientific Calculations
Apply
log10()
in a practical scenario, such as calculating the decibels of a sound intensity.javadouble intensity = 1000000; double referenceIntensity = 10; double decibels = 10 * Math.log10(intensity / referenceIntensity); System.out.println("Decibels: " + decibels);
This code calculates the decibels of a sound with an intensity of 1,000,000 relative to a reference intensity of 10. The output will be
50.0
, useful in fields like acoustics.
Conclusion
The Math.log10()
function in Java is essential for computing base-10 logarithms, critical in various scientific and statistical applications. By mastering log10()
, you enhance your ability to perform complex logarithmic calculations effortlessly and accurately. From handling basic positive numbers to dealing with zero and negative values, understanding the output of this function enables you to conduct precise logarithmic calculations under various circumstances. Incorporate these techniques to elevate the mathematical capabilities of your Java applications.
No comments yet.