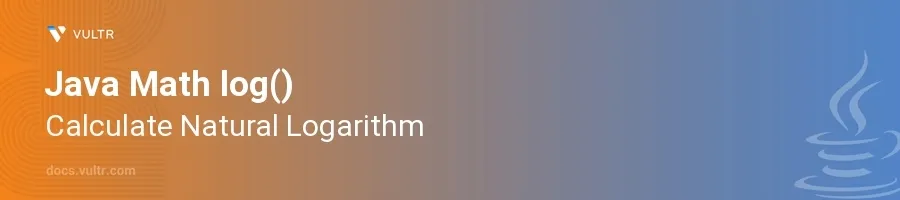
Introduction
The Math.log()
function in Java is essential for calculating the natural logarithm of a given value. This mathematical operation is fundamental in various fields like finance, science, and engineering where growth processes or time scaling are involved.
In this article, you will learn how to use the Math.log()
function in Java to calculate logarithms, handle special values, and apply it to real-world examples. Exploring different scenarios helps understand the behavior of logarithmic calculations in Java programming.
Using Math.log() for Basic Calculations
Calculate the Natural Logarithm of a Number
Ensure you import the Math class if your environment does not do so implicitly.
Pass a positive double value to
Math.log()
.javadouble result = Math.log(10); System.out.println("Natural logarithm of 10: " + result);
The code calculates the natural logarithm of 10. It outputs approximately 2.302, reflecting the power to which e (approx 2.71828) must be raised to produce 10.
Handle Negative Numbers and Zero
Recognize that the logarithm of zero or a negative number is undefined in the real number system.
Handle these cases appropriately in your code to avoid
NaN
or infinite results.javadouble logZero = Math.log(0); double logNegative = Math.log(-1); System.out.println("Logarithm of 0: " + logZero); System.out.println("Logarithm of -1: " + logNegative);
This snippet demonstrates that Java returns
-Infinity
for the log of zero andNaN
for the log of negative numbers.
Real-World Applications of Math.log()
Comparing Growth Rates
Use
Math.log()
to compare the growth rates of different processes.Calculate and interpret the logarithm of growth factors.
javadouble growthFactorA = 3.7; double growthFactorB = 2.3; double logA = Math.log(growthFactorA); double logB = Math.log(growthFactorB); System.out.println("Logarithmic growth rate of A: " + logA); System.out.println("Logarithmic growth rate of B: " + logB);
This code helps compare which process grows faster over time by examining their logarithms. Higher logarithmic value indicates faster growth.
Calculating Time Decay in Radioactive Materials
Apply the natural logarithm to determine the decay constant in radioactive decay formulas.
Implement the calculation in a real-world context by using
Math.log()
.javadouble remainingMaterial = 5; double initialMaterial = 10; double halfLife = Math.log(2) / Math.log(initialMaterial / remainingMaterial); System.out.println("Calculated half-life of material (in same time units as the decay rate): " + halfLife);
Here, the
Math.log()
function is crucial in deriving the half-life based on the ratio of the materials remaining and the initial amount, applying the natural logarithmic properties.
Conclusion
The Math.log()
function in Java enables efficient and accurate computations of natural logarithms which are integral in mathematical modeling, data analysis, and scientific research. By mastering the Math.log()
function, enhance the mathematical rigor of your Java applications to solve complex problems involving exponential growth and decay among others. Embrace the examples discussed as a foundation to extend the use in various domains requiring logarithmic calculations.
No comments yet.