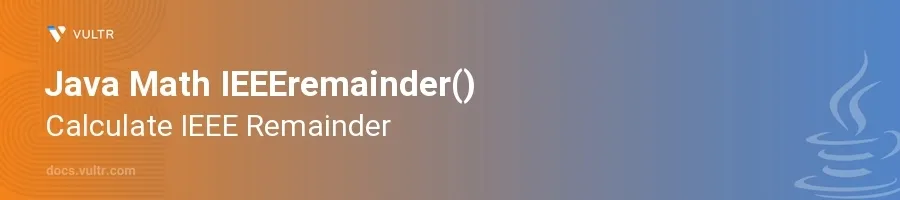
Introduction
The IEEEremainder()
function in Java falls under the Math class and provides an implementation for computing the remainder operation as per the IEEE 754 standard. This method is distinct in its approach as it bases the result on the nearest integer division. It's particularly useful in fields like computer graphics and numerical computation where precise and standardized mathematical operations are crucial.
In this article, you will learn how to effectively use the IEEEremainder()
method to calculate remainders in compliance with the IEEE 754 standard. You’ll explore its behavior with various examples and see how it differs from the traditional remainder calculation methods.
Understanding IEEEremainder() in Java
Basics of the IEEEremainder Function
IEEE 754 standard dictates that the remainder should be the difference between the dividend and the closest product of the divisor and an integer. The IEEEremainder()
function helps achieve exactly that.
Review the method signature: The
IEEEremainder()
function takes two double parameters: the dividend (f1
) and the divisor (f2
). It returns the remainder as a double.Know the syntax:
javadouble result = Math.IEEEremainder(double f1, double f2);
Example Calculations
Calculate the IEEE Remainder of Two Numbers
Define two double variables for the dividend and divisor.
Call the
IEEEremainder()
method and store the result.Print the result.
javadouble dividend = 23.5; double divisor = 5.5; double result = Math.IEEEremainder(dividend, divisor); System.out.println("IEEE Remainder of 23.5 % 5.5: " + result);
This code calculates the IEEE remainder when 23.5 is divided by 5.5 and prints the result. The function calculates the nearest integer multiple of 5.5 to 23.5, determines the difference, and thus, derives the remainder.
Compare IEEEremainder() with Normal Modulus Operator
Calculate the remainder using both
IEEEremainder()
and the modulus operator%
.Output both results to compare.
javadouble normalRemainder = 23.5 % 5.5; double ieeeRemainder = Math.IEEEremainder(23.5, 5.5); System.out.println("Normal remainder: " + normalRemainder); System.out.println("IEEE remainder: " + ieeeRemainder);
This example highlights the difference in results between the traditional modulus and the IEEE remainder. The IEEE method provides a result that adheres to the rounding standard of getting the nearest divisor product.
Common Use Cases and Benefits
- Graphics and Simulations: Utilize
IEEEremainder()
for error corrections in periodic conditions in graphics programming or simulations where accuracy is crucial. - Data Analysis: Ensure standardized computations in data analysis processes where IEEE 754 compliance is required for cross-platform consistency.
- Scientific Computing: Apply
IEEEremainder()
in situations where the exactness of mathematical calculations impacts the outcomes significantly.
Conclusion
The IEEEremainder()
function in Java is a powerful tool for computing remainders strictly according to the IEEE 754 standard. Its precision and compliance make it essential for tasks requiring accurate and consistent mathematical results. By providing mechanisms to calculate the remainder based on the nearest integer, it helps enhance the accuracy of your computations significantly. Use it to maintain standardization across various computational tasks, especially where minute differences can lead to major discrepancies in outcomes. With the examples and scenarios provided, leverage this function to streamline your solutions in various applications that demand high arithmetic precision.
No comments yet.