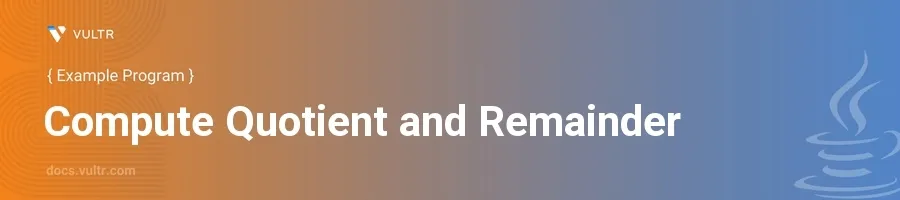
Introduction
Java is a versatile programming language that is widely used for building diverse applications, from web applications to mobile Android apps. Among its many capabilities, performing basic arithmetic operations is foundational in Java. At the core of many mathematical computations are the operations to find the quotient and the remainder, commonly used in algorithms that involve division.
In this article, you will learn how to create a Java program to calculate the quotient and the remainder when one number is divided by another. The examples provided will guide you through various scenarios, demonstrating the use of the division and modulus operators.
Computing Quotient and Remainder in Java
Basic Example
Start by defining two integer variables to hold the dividend and the divisor.
Use the division
/
operator to compute the quotient and the modulus%
operator to compute the remainder.Print the results using
System.out.println
.javapublic class Main { public static void main(String[] args) { int dividend = 25; int divisor = 4; int quotient = dividend / divisor; int remainder = dividend % divisor; System.out.println("Quotient: " + quotient); System.out.println("Remainder: " + remainder); } }
This program divides 25 by 4. It calculates the quotient as
6
and the remainder as1
.
Handling Zero as a Divisor
Include a condition to check whether the divisor is zero before performing division.
Implement error handling to avoid
ArithmeticException
.Output a message if the divisor is zero to inform the user.
javapublic class Main { public static void main(String[] args) { int dividend = 25; int divisor = 0; // set divisor as zero to demonstrate error handling if (divisor != 0) { int quotient = dividend / divisor; int remainder = dividend % divisor; System.out.println("Quotient: " + quotient); System.out.println("Remainder: " + remainder); } else { System.out.println("Divisor cannot be zero."); } } }
This code ensures that the division operation does not proceed if the divisor is zero, thus avoiding a runtime error.
Using Scanner for User Input
Import the
Scanner
class for user input.Prompt the user to enter the dividend and the divisor.
Compute the quotient and remainder as before and display the results.
javaimport java.util.Scanner; public class Main { public static void main(String[] args) { Scanner scanner = new Scanner(System.in); System.out.println("Enter the dividend:"); int dividend = scanner.nextInt(); System.out.println("Enter the divisor:"); int divisor = scanner.nextInt(); if (divisor != 0) { int quotient = dividend / divisor; int remainder = dividend % divisor; System.out.println("Quotient: " + quotient); System.out.println("Remainder: " + remainder); } else { System.out.println("Divisor cannot be zero."); } scanner.close(); } }
This program dynamically accepts user input for the dividend and divisor, then calculates and displays the quotient and remainder.
Conclusion
Calculating the quotient and remainder is a fundamental skill in Java programming that finds applications in many areas such as mathematical algorithms, digital systems, and more. The examples discussed serve as a primer to dealing with basic division, error handling, and incorporating user input. As you develop more complex Java applications, understanding these operations will enhance your ability to solve problems effectively and efficiently.
No comments yet.