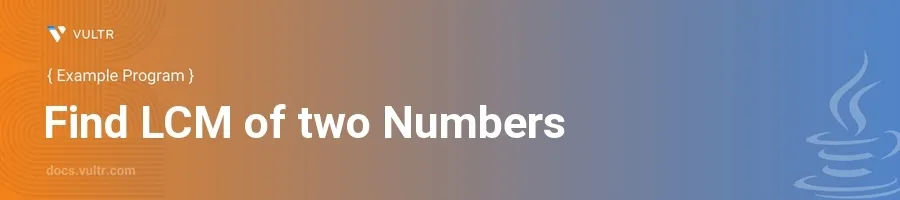
Introduction
The Least Common Multiple (LCM) of two numbers is the smallest number that is a multiple of both. Calculating the LCM is a common task in various fields such as mathematics, engineering, and computer science for solving problems involving fractions, ratios, and periodic events. Understanding how to compute the LCM is essential for optimizing algorithms and ensuring efficient calculations in performance-critical applications.
In this article, you will learn how to develop a Java program to find the LCM of two numbers. You'll see the implementation of two distinct methods: using the Greatest Common Divisor (GCD) approach, and an efficient algorithm that avoids the GCD computation. Through step-by-step examples, grasp the practical application and comparative efficiency of these approaches.
Finding LCM Using GCD
The LCM of two integers can be found using the relationship between LCM and GCD (Greatest Common Divisor). The formula to find the LCM using GCD is:
[ \text{LCM}(a, b) = \frac{|a \times b|}{\text{GCD}(a, b)} ]
Java Implementation
First, create a function to compute the GCD using the Euclidean algorithm.
Then, use the GCD to compute the LCM as per the formula.
javapublic class LCMFinder { public static int gcd(int a, int b) { while (b != 0) { int t = b; b = a % b; a = t; } return a; } public static int lcm(int a, int b) { return (a / gcd(a, b)) * b; // Prevent potential overflow } public static void main(String[] args) { int num1 = 12; int num2 = 15; System.out.println("LCM of " + num1 + " and " + num2 + " is " + lcm(num1, num2)); } }
This code calculates the LCM of two numbers,
12
and15
. It first calculates the GCD of these numbers and then applies the formula to find the LCM, which in this case is60
.
Direct LCM Calculation Without GCD
It's also possible to calculate the LCM by directly comparing multiples of the two numbers. This method is less efficient but instructive for understanding how LCM works at a fundamental level.
Java Implementation
Initialize a value to the maximum of the two numbers.
Increment this value until it is a multiple of both numbers.
javapublic class LCMFinderDirect { public static int lcmDirect(int a, int b) { int max = Math.max(a, b); int lcm = max; while (true) { if (lcm % a == 0 && lcm % b == 0) { break; } lcm += max; } return lcm; } public static void main(String[] args) { int num1 = 12; int num2 = 15; System.out.println("LCM (Direct Method) of " + num1 + " and " + num2 + " is " + lcmDirect(num1, num2)); } }
Here, the LCM of
12
and15
is computed by incrementally checking each multiple of the larger number (15
in this case) until a common multiple is found.
Conclusion
Understanding different methods to compute the LCM in Java offers practical insights into mathematical algorithms and their optimizations. Whether using the GCD-based approach for efficiency or the direct multiple comparison for educational purposes, these methods provide a strong foundation in both theoretical and applied computing concepts. Implement these methods in Java to enhance your problem-solving skills and optimize your mathematical computations in software development.
No comments yet.