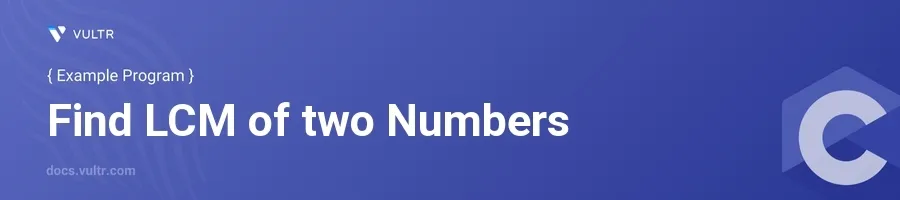
Introduction
The Least Common Multiple (LCM) of two numbers is the smallest number that is a multiple of both. It's a concept widely used in arithmetic and number theory and has practical applications in problems involving work completion times, synchronization of events, and more. Computing the LCM gives insights into periodicity and alignment in various mathematical and real-world scenarios.
In this article, you will learn how to compute the LCM of two numbers in C programming through detailed examples. Discover how to apply foundational arithmetic operations such as division, multiplication, and the use of the Greatest Common Divisor (GCD) to achieve this, deepening your understanding of algorithmic solutions in C.
Basic Algorithm for LCM Calculation
Using GCD to Find LCM
Understand the relationship between GCD and LCM:
LCM(a, b) * GCD(a, b) = a * b
.Write a function to compute the GCD using the Euclidean algorithm.
Implement the LCM function utilizing the GCD function.
c#include <stdio.h> int gcd(int a, int b) { while (b != 0) { int t = b; b = a % b; a = t; } return a; } int lcm(int a, int b) { return (a / gcd(a, b)) * b; } int main() { int num1 = 12, num2 = 18; printf("LCM of %d and %d is %d\n", num1, num2, lcm(num1, num2)); return 0; }
This code defines two functions,
gcd()
andlcm()
.gcd()
computes the Greatest Common Divisor using the Euclidean algorithm, andlcm()
calculates the Least Common Multiple using the relation between LCM and GCD.
Handling Zero Inputs
Recognize that the mathematical property when one of the numbers is zero,
LCM(0, b) = 0
andLCM(a, 0) = 0
, because zero multiplied by any number is zero.Modify the
lcm()
function to handle zero as input effectively.cint lcm(int a, int b) { if (a == 0 || b == 0) { return 0; } return (a / gcd(a, b)) * b; }
With this modification, the
lcm()
function now correctly returns 0 when either of the input numbers is zero, ensuring adherence to mathematical rules.
Conclusion
Calculating the LCM of two numbers in C utilizing the GCD method is a fundamental yet powerful approach in computational number theory. By analyzing the relational formula that connects both concepts, you ensure efficient and mathematically sound computation. Overall, understanding and implementing algorithms for foundational mathematical operations like LCM not only enhances your problem-solving skills but also deepens your proficiency with the C programming language.
No comments yet.