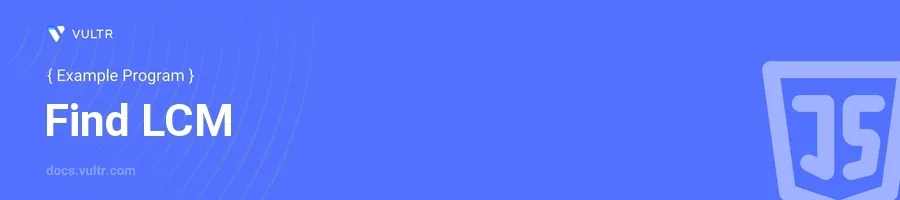
Introduction
Finding the Least Common Multiple (LCM) of two numbers is a common task in mathematical computations and programming. The LCM of two integers is the smallest positive integer that is divisible by both. It's essential for solving problems that involve addition or subtraction of fractions with different denominators or arranging events that cycle at differing intervals.
In this article, you will learn how to efficiently compute the LCM of two numbers using JavaScript. Discover how to handle this calculation through practical and simple examples, providing you with the tools to apply these methods in various programming contexts.
Finding LCM Using Iteration
Understand the Concept with an Iterative Approach
Start with the higher of the two numbers as the possible LCM.
Incrementally test whether this number is divisible by both input numbers.
javascriptfunction findLCM(num1, num2) { let max = Math.max(num1, num2); let lcm = max; while (true) { if (lcm % num1 === 0 && lcm % num2 === 0) { break; } lcm += max; } return lcm; } console.log(findLCM(4, 6)); // Output: 12
In this example, the function
findLCM
starts checking from the highest of the two numbers (num1
andnum2
). It continues to increment the candidate LCM by this max value until it finds a number that is divisible by bothnum1
andnum2
. The first such number is the LCM.
Apply Iteration in a Practical Scenario
Consider a scenario where you have two processes with different cycle times, and you need to find when they will coincide.
javascriptfunction coincidingTime(processA, processB) { return findLCM(processA, processB); } console.log(coincidingTime(24, 36)); // Output: 72
This snippet uses the previously defined
findLCM
function. IfprocessA
has a cycle of 24 units, andprocessB
has a cycle of 36 units, this function will calculate when both processes coincide in time, which can be handy for scheduling or synchronization tasks.
Finding LCM Using the GCD Method
Efficient Calculation with the GCD (Greatest Common Divisor)
Employ the mathematical relationship, LCM(a, b) = |a * b| / GCD(a, b), to compute the LCM, using the Euclidean algorithm for finding the GCD.
javascriptfunction gcd(a, b) { while (b !== 0) { let t = b; b = a % b; a = t; } return a; } function lcmUsingGCD(num1, num2) { return Math.abs(num1 * num2) / gcd(num1, num2); } console.log(lcmUsingGCD(8, 12)); // Output: 24
The Euclidean algorithm recursively reduces the problem of finding the GCD of two numbers until the remainder is zero. The LCM is then calculated using the absolute product of the two numbers divided by their GCD.
Use the GCD Method in Complex Calculations
Extend the method to find the LCM of multiple numbers in an array, which can be useful in more complex scenarios involving several different recurring cycles.
javascriptfunction lcmOfArray(arr) { return arr.reduce((lcm, current) => lcmUsingGCD(lcm, current), 1); } console.log(lcmOfArray([4, 8, 14])); // Output: 56
This function utilizes
lcmUsingGCD
to iteratively calculate the LCM of an entire array of numbers, significantly simplifying the process when dealing with multiple elements.
Conclusion
Computing the LCM in JavaScript can be tackled using either iterative or GCD-based approaches, each suitable for different situations depending on the complexity and the performance needs. The mathematical foundation behind these methods not only enhances your understanding but also improves how you implement solutions involving numerical computations in JavaScript. Apply these techniques as shown to effectively manage any challenges that require finding the least common multiple in both simple and complex environments.
No comments yet.