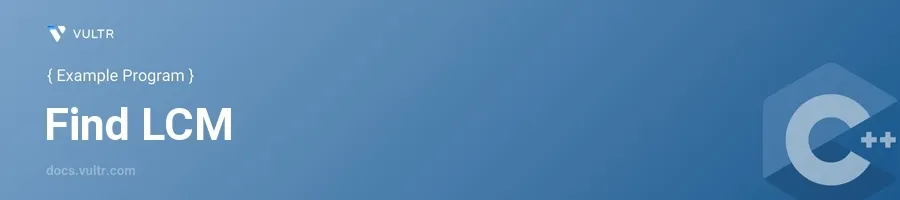
Introduction
The least common multiple (LCM) of two integers is the smallest number that is a multiple of both integers. In computer programming, particularly in C++, computing the LCM is often required for solving problems related to number theory or where synchronization of cycles occurs. It’s a concept frequently utilized in algorithm design and optimization.
In this article, you will learn how to create a C++ program to find the least common multiple of two numbers using several approaches. Each method will be accompanied by examples, segmenting the otherwise daunting task into manageable, easy-to-understand components. Understand not just the code, but the mechanics behind these calculations, enhancing your problem-solving skills in C++.
Calculating LCM using the GCD Approach
Step 1: Understanding the Relationship Between GCD and LCM
- Recognize that LCM of two numbers can be found using their greatest common divisor (GCD).
- Recall the formula: [ \text{LCM}(a, b) = \left|\frac{a \times b}{\text{GCD}(a, b)}\right| ]
Step 2: Implementation Using C++
Implement the GCD function using Euclidean algorithm.
Invoke this function to calculate the LCM.
cpp#include <iostream> using namespace std; int gcd(int a, int b) { while (b != 0) { int t = b; b = a % b; a = t; } return a; } int lcm(int a, int b) { int h = gcd(a, b); return abs(a * (b / h)); } int main() { int num1, num2; cout << "Enter two numbers: "; cin >> num1 >> num2; cout << "LCM of " << num1 << " and " << num2 << " is " << lcm(num1, num2) << endl; return 0; }
In this code, the
gcd
function first determines the greatest common divisor of the two input numbers using the Euclidean algorithm. Thelcm
function then calculates the Least Common Multiple based on the formula mentioned above. The main function facilitates user input and displays the output.
Using Iterative Approach to Find LCM
Step 1: Brute Force Method
- Understand that LCM can be computed by increasing increments of the larger number until it becomes a multiple of the smaller number.
Step 2: Implement Brute Force in C++
Use loops to incrementally check every multiple of the larger number.
cpp#include <iostream> using namespace std; int lcm(int a, int b) { int max = (a > b) ? a : b; while (true) { if ((max % a == 0) && (max % b == 0)) { break; } ++max; } return max; } int main() { int num1, num2; cout << "Enter two numbers: "; cin >> num1 >> num2; cout << "LCM of " << num1 << " and " << num2 << " is " << lcm(num1, num2) << endl; return 0; }
This snippet demonstrates a more straightforward, though less efficient, way of determining the LCM by brute force method. The function iterates through multiples of the greater of the two numbers, testing each one to see if it's divisible by both integers.
Conclusion
The computation of LCM in C++ can be done using various methods including leveraging the GCD or using a more intuitive iterative approach. This ability to compute the least common multiple effectively participates in solving a wide array of problems in computer science and mathematics. By mastering these two techniques, you bolster your ability to handle numerical computations and algorithmic challenges in C++.
No comments yet.