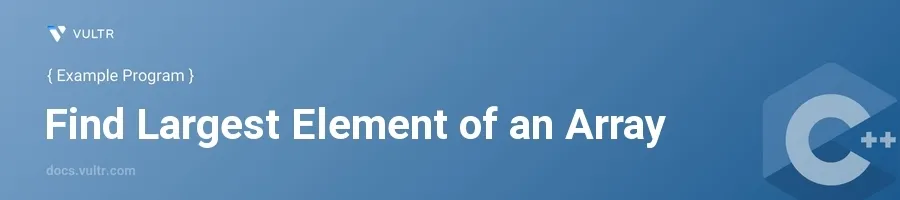
Introduction
When working with arrays in C++, a common task you might encounter is finding the largest element in the array. This operation is fundamental in various contexts, such as data analysis, statistics, and algorithm optimization. Understanding how to efficiently find the maximum value in an array can significantly influence the performance and scalability of your applications.
In this article, you will learn how to find the largest element in an array using C++. We'll explore different methods including a basic loop method, the use of standard library functions, and a comparison against a recursive approach, each illustrated with clear examples to help you understand and implement these solutions effectively in your C++ projects.
Basic Loop Method
Finding the largest element in an array using a basic loop involves iterating through each element, maintaining a variable that holds the maximum value found at each step. This method is straightforward and doesn't require any additional libraries.
Example Using Basic Loop
Define an array of integers.
Initialize a variable to store the maximum value, starting with the first element of the array.
Use a loop to iterate through the array elements from the second position and update the maximum value if a larger number is found.
cpp#include <iostream> using namespace std; int main() { int array[] = {3, 57, 12, 98, 32}; int maxVal = array[0]; // Start with the first element for(int i = 1; i < 5; i++) { if(array[i] > maxVal) { maxVal = array[i]; } } cout << "The largest element in the array is " << maxVal << endl; return 0; }
This code maintains a
maxVal
variable that captures the largest value encountered during the iteration through the array, ultimately printing the largest value found.
Utilizing Standard Library Functions
The C++ Standard Library provides powerful functions that can simplify common tasks, such as finding the maximum element in an array. The function std::max_element
from the <algorithm>
header is specifically designed for this purpose.
Example Using std::max_element
Include the
<algorithm>
and<iterator>
headers that contain the necessary functions.Use the
std::max_element
function to find the iterator to the largest element.Dereference the iterator to get the value of the maximum element.
cpp#include <iostream> #include <algorithm> // Include for std::max_element #include <iterator> // Include for std::begin, std::end int main() { int array[] = {3, 57, 12, 98, 32}; // Use std::max_element to find the largest element int* maxElementPtr = std::max_element(std::begin(array), std::end(array)); cout << "The largest element in the array is " << *maxElementPtr << endl; return 0; }
Here,
std::max_element
is used to find the largest element by comparing all array elements. The function returns an iterator pointing to the maximum element.
Recursive Method: An Alternative Approach
If you prefer a recursive approach to solve problems, particularly for academic or intellectual curiosity, recursion can be used to find the largest element in an array.
Example Using Recursion
Define a recursive function that compares the current element with the maximum of remaining elements.
Use this function to find the largest element.
cpp#include <iostream> using namespace std; int findMaxRecursively(int array[], int n) { // Base case: If array has only one element if (n == 1) return array[0]; // Recursive call to process the rest of the array return max(array[n-1], findMaxRecursively(array, n-1)); } int main() { int array[] = {3, 57, 12, 98, 32}; int n = sizeof(array)/sizeof(array[0]); cout << "The largest element in the array is " << findMaxRecursively(array, n) << endl; return 0; }
In this recursive method, the function
findMaxRecursively
recursively breaks down the problem into smaller sub-problems until it reaches the base case of a single element.
Conclusion
Finding the largest element in an array using C++ offers various methods each suited to different situations and preferences. From a simple loop-based approach to utilizing potent standard library functions, or even applying a recursive solution, you have flexibility in your approach based on the requirements of the problem and your personal coding style. Expand your understanding and use these examples to enhance your ability to work with arrays in C++.
No comments yet.