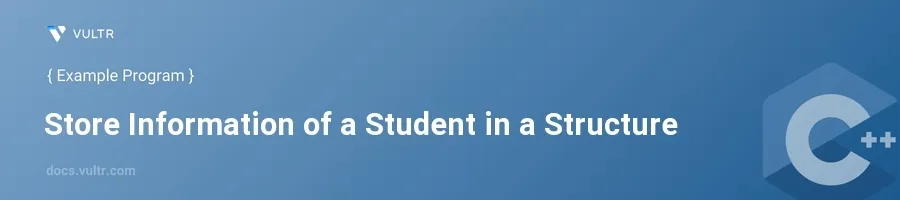
Introduction
In C++, structures provide a way to group different data types together under a single unit. This characteristic makes them ideal for storing complex data such as the information of a student, which typically includes name, age, marks, and other attributes. In programming constructs, structures can significantly streamline handling related data and enhance code organization.
In this article, you will learn how to utilize structures in C++ to store and manipulate data related to a student. Discover the step-by-step process of declaring a structure, setting values to its members, and accessing these values efficiently. By the end of this guide, manage student data with ease using C++ structures in practical examples.
Defining a Student Structure
Create the Structure
Begin by defining a structure named
Student
.Include members like
name
,age
, and an arraymarks
to store scores in different subjects.cppstruct Student { char name[50]; int age; int marks[5]; // Assumes marks for 5 subjects };
This code snippet declares a
Student
structure with three fields.name
stores the student's name,age
keeps the age, andmarks
is an array that holds the scores for five subjects.
Initialize a Student Instance
Create a variable of type
Student
.Assign values to the fields
name
,age
, andmarks
.cppStudent student1; strcpy(student1.name, "John Doe"); // Copying string into char array student1.age = 20; int sample_marks[5] = {87, 78, 93, 85, 90}; for (int i = 0; i < 5; i++) { student1.marks[i] = sample_marks[i]; }
In this code,
student1
is instantiated as aStudent
. The string copy functionstrcpy
is used to set the name due to the C-style string in array format. Then,age
andmarks
for five subjects are set directly.
Accessing Structure Members
Reading Data from a Structure
Access the individual members of the structure using the dot operator.
cppcout << "Name: " << student1.name << endl; cout << "Age: " << student1.age << endl; cout << "Marks: "; for (int mark : student1.marks) { cout << mark << " "; } cout << endl;
This snippet prints the data stored in
student1
. It uses a range-based for loop to iterate over the arraymarks
. This style of loop simplifies accessing array elements without needing index variables.
Sharing Structures Between Functions
Passing Structure to a Function
Define a function that accepts a
Student
structure as a parameter to display its contents.Call the function, passing the structure as an argument.
cppvoid printStudentInfo(const Student& stu) { cout << "Student Information:\n"; cout << "Name: " << stu.name << endl; cout << "Age: " << stu.age << endl; cout << "Marks: "; for (int mark : stu.marks) { cout << mark << " "; } cout << endl; } printStudentInfo(student1);
The function
printStudentInfo
takes aStudent
structure as a reference to prevent copying the entire structure (which could be costly with larger structures). It then prints all the elements of the structure in a formatted style.
Updating Structure Information
Modify Members of a Structure
Update specific fields in the
Student
structure to reflect data changes.Display the updated information.
cppstrcpy(student1.name, "Jane Smith"); // Update name student1.age = 22; // Update age int updated_marks[5] = {91, 81, 88, 92, 95}; for (int i = 0; i < 5; i++) { student1.marks[i] = updated_marks[i]; } printStudentInfo(student1);
This modifies values in
student1
, changing the name, age, and marks. After updating, use the sameprintStudentInfo
function to display the new data.
Conclusion
Utilizing structures in C++ to encapsulate and manage data, like student information, enhances modularity and readability of your code. By creating the Student
structure, you can efficiently handle related attributes and perform operations on them. This guide not only demonstrates the initial setup but also updating and accessing the data with structures. Apply these practices to maintain highly organized code especially when dealing with various complex data types in larger software development projects.
No comments yet.