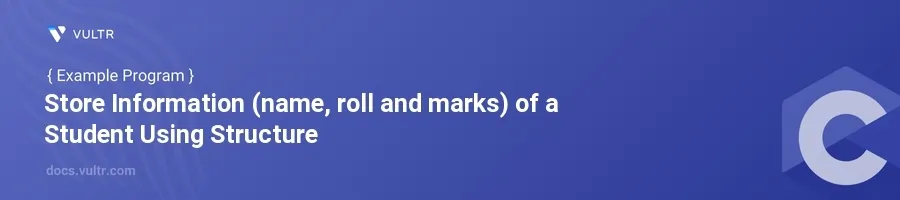
Introduction
Working with complex data types in the C programming language often involves creating structured forms of data collections. One of the most valuable data types in C for managing related data is the structure (struct
). A structure allows you to store different data types together under a single umbrella. This is especially useful for organizing records like student information, which includes a name, roll number, and marks.
In this article, you will learn how to write a C program to display student details using structures. Discover how to define a structure, store data in it, and display this information, all using clear examples to guide you. You will also learn how to write a program that stores and prints the roll number, name, age, and marks of a student using structures.
Structure Program for Student Details in C
Creating the Student Structure
Define a structure named
Student
to hold a student's name, roll number, and marks.Include the standard input-output library header
stdio.h
for input and output operations.c#include <stdio.h> struct Student { char name[50]; int roll; float marks; };
This block of code defines a new structure type called
Student
, which can now be used to create variables that can store a student's name, roll number, and marks. The name is stored as an array of characters.
C Program to Store Information of Students by Inputting Data into the Structure
Prompt User Input to Fill the Structure
Declare a
Student
variable.Prompt the user to enter the details of the student.
cint main() { struct Student student1; printf("Enter student's name: "); fgets(student1.name, sizeof(student1.name), stdin); printf("Enter roll number: "); scanf("%d", &student1.roll); printf("Enter marks: "); scanf("%f", &student1.marks); return 0; }
In this code,
fgets
is used to read a string input, which includes spaces, from the user. Thescanf
function reads the integer and float values for the roll number and marks. Note the use of&
inscanf
for numerical inputs to provide the address where the inputted value will be stored.
C Program to Display Student Details Using Structure
Output the Data Stored in the Structure
Print out the information stored in the structure to verify correct input.
cint main() { struct Student student1; // Input code here as previously shown printf("\nStudent's Name: %s", student1.name); printf("Roll Number: %d\n", student1.roll); printf("Marks: %.2f\n", student1.marks); return 0; }
This snippet outputs the data entered by the user. The use of
%.2f
in theprintf
statement for displaying marks formats the float to two decimal places, enhancing the readability of the output.
Structure Program for Student Details in C using Function
C program that uses a struct to store student details and functions to input and display the details.
Uses a struct to store student details (Name, Roll Number, Marks).
Includes functions to input and display student details.
Supports multiple students using an array.
cstruct Student { char name[50]; int rollNumber; float marks; }; void inputStudentDetails(struct Student *s) { printf("Enter Name: "); scanf(" %[^\n]", s->name); printf("Enter Roll Number: "); scanf("%d", &s->rollNumber); printf("Enter Marks: "); scanf("%f", &s->marks); } void displayStudentDetails(struct Student s) { printf("\nStudent Details:\n"); printf("Name: %s\n", s.name); printf("Roll Number: %d\n", s.rollNumber); printf("Marks: %.2f\n", s.marks); } int main() { int n; printf("Enter the number of students: "); scanf("%d", &n); struct Student students[n]; for (int i = 0; i < n; i++) { printf("\nEnter details for student %d:\n", i + 1); inputStudentDetails(&students[i]); } for (int i = 0; i < n; i++) { displayStudentDetails(students[i]); } return 0; }
Explanation:
Structure Definition (struct Student): Contains name, rollNumber, and marks.
inputStudentDetails() Function: Takes user input and fills the structure.
displayStudentDetails() Function: Prints student details.
Main Function (main()): 4.1. Accepts the number of students. 4.2. Loops through to input details for each student. 4.3. Displays all student details.
Conclusion
Understanding and using structures in C programming enable efficient management of grouped data. In this guide, by defining a Student structure, you learned to input and display complex and varied data types cohesively. This approach is fundamental when dealing with records or collections of related information. Apply these concepts to create structures for different data handling scenarios to keep your programs organized and maintainable. Through these structures, your code not only becomes cleaner but also easier to manage and scale.
No comments yet.