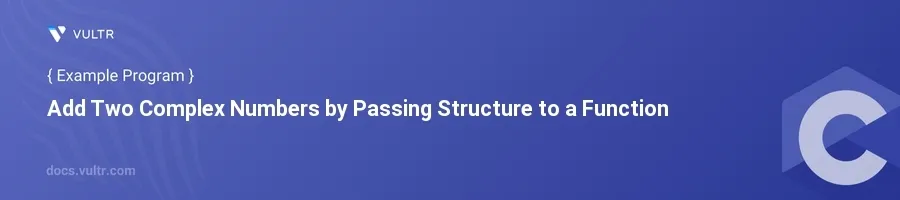
Introduction
Working with complex numbers is a fundamental concept in various scientific and engineering disciplines. In programming, handling complex numbers efficiently can lead to more robust and readable code, especially in languages like C where you need to manage data structures manually. This article explores how to create and manipulate complex numbers using structures in C by passing them to a function that performs addition.
In this article, you will learn how to define a structure for complex numbers in C, how to add two complex numbers by passing these structures to a function, and finally, analyze a few examples that demonstrate the application of these concepts.
Defining the Complex Number Structure
Creating a structure for a complex number allows you to encapsulate both the real and imaginary parts of the number into a single entity. This makes it easier to manage and pass complex numbers to functions.
Define the structure for a complex number.
ctypedef struct { float real; float imag; } Complex;
This code defines a
Complex
type with twofloat
members:real
andimag
, which represent the real and imaginary parts of the complex number, respectively.
Adding Two Complex Numbers
To add two complex numbers, you need a function that takes two Complex
structures as arguments and returns a Complex
structure as the result.
Write a function to add two complex numbers.
cComplex addComplexNumbers(Complex a, Complex b) { Complex result; result.real = a.real + b.real; result.imag = a.imag + b.imag; return result; }
This function takes two
Complex
structures,a
andb
, adds their real and imaginary parts separately, and returns the resultant complex number.
Examples
Putting it all together, you can now write a main function to demonstrate adding two complex numbers using the defined structure and function.
Implement the main function to add complex numbers.
c#include <stdio.h> int main() { Complex num1, num2, sum; num1.real = 3.0; num1.imag = 2.0; num2.real = 1.0; num2.imag = 7.0; sum = addComplexNumbers(num1, num2); printf("Sum = %.1f + %.1fi\n", sum.real, sum.imag); return 0; }
In this example,
num1
andnum2
are initialized with specific real and imaginary values. TheaddComplexNumbers
function is called with these numbers, and the resulting complex number is stored insum
. The result is then printed to the console.
Conclusion
By defining a structure for complex numbers and utilizing functions that operate on these structures, you can manage complex numbers effectively in C programming. The method demonstrated not only keeps the code clean and organized but also modularizes the functionality, allowing for easier maintenance and understanding. Remember to utilize structures and function passing effectively when dealing with similar data-centric tasks in C.
No comments yet.