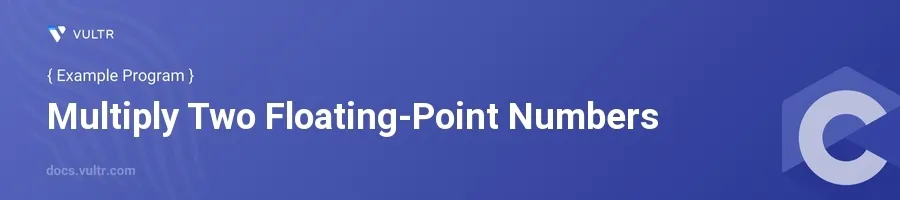
Introduction
Multiplying two floating-point numbers in C is a fundamental operation that's common in many types of software applications, ranging from scientific computing to game development. Floating-point arithmetic allows for the representation of real numbers that require precision over wide ranges.
In this article, you will learn how to effectively multiply two floating-point numbers in C. You will explore several examples that illustrate the process, ensuring you have the knowledge to implement this operation in various contexts effectively.
Basic Multiplication of Two Floats
Performing a Simple Multiplication
Declare and initialize two floating-point variables.
Multiply these variables and store the result in another floating-point variable.
Output the result using
printf
.c#include <stdio.h> int main() { float a = 3.5f; float b = 4.2f; float result = a * b; printf("The result of %.2f multiplied by %.2f is %.2f\n", a, b, result); return 0; }
This code initializes two floats
a
andb
, multiplies them, and stores the result inresult
. Finally, it prints the result.%f
in theprintf
function is used to format floating-point numbers, and%.2f
specifies that two decimal places should be displayed.
Handling Precision
Work with double precision floats if higher accuracy is necessary.
Perform the multiplication similarly to the above example, but use the
double
type.c#include <stdio.h> int main() { double a = 3.5467; double b = 4.2135; double result = a * b; printf("The result of %.4f multiplied by %.4f is %.4f\n", a, b, result); return 0; }
Using
double
instead offloat
improves the precision of the calculations. In this example, four decimal places are displayed.
Advanced Multiplications
Multiplying Arrays of Floating-Point Numbers
Use arrays to store multiple floating-point numbers.
Multiply elements from two arrays in a loop.
c#include <stdio.h> int main() { float nums1[] = {2.5f, 3.5f, 4.5f}; float nums2[] = {1.5f, 2.5f, 3.5f}; int length = sizeof(nums1) / sizeof(nums1[0]); float results[length]; for (int i = 0; i < length; i++) { results[i] = nums1[i] * nums2[i]; printf("%.2f * %.2f = %.2f\n", nums1[i], nums2[i], results[i]); } return 0; }
This snippet multiplies each element of
nums1
with the corresponding element innums2
and stores each result in theresults
array. It uses a loop to process all elements.
Conclusion
Multiplying floating-point numbers in C is straightforward but requires attention to detail regarding types and precision. From simple operations to multiplying arrays, understanding these principles ensures accurate and efficient mathematical computations in your C programs. Implementing these examples will solidify your foundation in handling floating-point arithmetic in various programming challenges.
No comments yet.