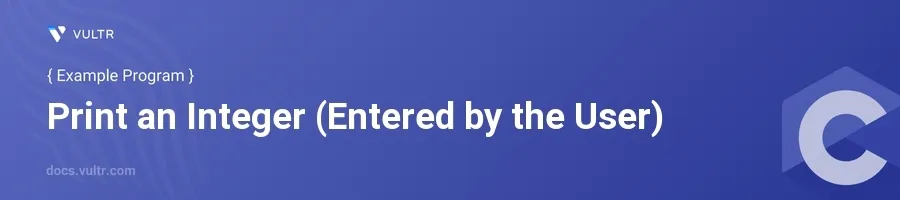
Introduction
In C programming, accepting user input and displaying it back to the user is a fundamental concept implemented in many beginner projects and applications. This basic operation helps new programmers understand how to interact with users effectively and process data within their programs.
In this article, you will learn how to create a simple C program that prompts a user to enter an integer and then prints that integer on the screen. Explore hands-on examples to solidify your understanding of handling user input and output in C.
Creating the Program
Prompt the User for an Integer
Begin by including the necessary header files.
stdio.h
is essential because it contains declarations for input/output functions.Declare a main function which is the entry point of any C program.
Inside the main function, declare an integer variable to store the user input.
Use
printf
function to prompt the user to enter an integer.Utilize
scanf
to read and store the user-input integer.c#include <stdio.h> int main() { int number; printf("Enter an integer: "); scanf("%d", &number); }
This code snippet initializes a variable
number
and then usesprintf
to provide a prompt.scanf
with%d
reads an integer from the input and stores it in the variablenumber
.
Display the Entered Integer
After storing the input, use
printf
again to display the entered integer.End the main function with a return statement to exit the program.
c#include <stdio.h> int main() { int number; printf("Enter an integer: "); scanf("%d", &number); printf("You entered: %d", number); return 0; }
Here, the entered integer is displayed using
printf
where%d
formats the integer for output. The program then ends withreturn 0
, signaling successful execution.
Conclusion
The C program demonstrated above effectively gathers an integer input from the user and displays it. This basic pattern is fundamental in learning C programming, as it combines both input and output operations which are essential in many applications. Follow these examples to create robust programs that can interact with users by accepting and processing user inputs efficiently.
No comments yet.