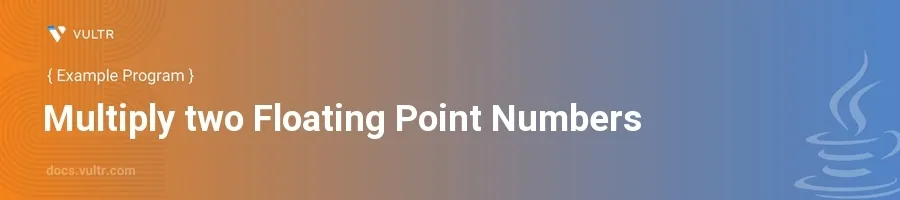
Introduction
Multiplying two floating-point numbers in Java is a basic yet crucial operation in many computational programs, from financial calculations to scientific computing. Java handles these operations with precision using the float
or double
data types, which accommodate the decimal and exponential parts of values.
In this article, you will learn how to perform multiplication of two floating-point numbers in Java through straightforward code examples. The techniques demonstrated will lay the groundwork for incorporating such operations into larger, more complex Java applications. By exploring a Java program to multiply two floating-point numbers, you'll gain practical insights into handling arithmetic operations with precision in Java.
Basic Multiplication of Two Floating-Point Numbers
Single Precision Using float
Declare two
float
variables.Multiply the variables and store the result.
Print the result.
javapublic class FloatMultiplication { public static void main(String[] args) { float number1 = 2.5f; float number2 = 4.2f; float product = number1 * number2; System.out.println("Product of two floating point numbers: " + product); } }
In this example,
number1
andnumber2
are multiplies, and the product is printed. Thef
suffix in the float literals specifies that they arefloat
data types.
Double Precision Using double
Declare two
double
variables.Multiply these variables.
Output the result.
javapublic class DoubleMultiplication { public static void main(String[] args) { double number1 = 2.523; double number2 = 4.234; double product = number1 * number2; System.out.println("Product of two floating point numbers: " + product); } }
This code block uses the java double
multiplication approach for better precision in calculations. The result of the multiplication is stored in product
and then displayed.
Handling Edge Cases
Consider multiplying very large or very small numbers.
Check for multiplication result regularities or exceptions like overflow or underflow.
javapublic class EdgeCaseMultiplication { public static void main(String[] args) { double largeNumber = 1e307; double smallNumber = 1e-307; double product = largeNumber * smallNumber; System.out.println("Product of edge case numbers: " + product); } }
Testing with extreme values such as
1e307
(a number near the upper limit ofdouble
) and1e-307
(very close to zero) illustrates how Java handles unusual cases, such as potential overflows or underflows without throwing errors.
Conclusion
Multiplying two floating-point numbers in Java is straightforward, whether using float
for standard precision or double
for higher precision. The examples provided show how to perform simple multiplications and address potential complications with extreme numerical values. By understanding how to multiply two numbers in Java, you can apply these principles in your applications to handle numerical computations with confidence and correctness.
No comments yet.