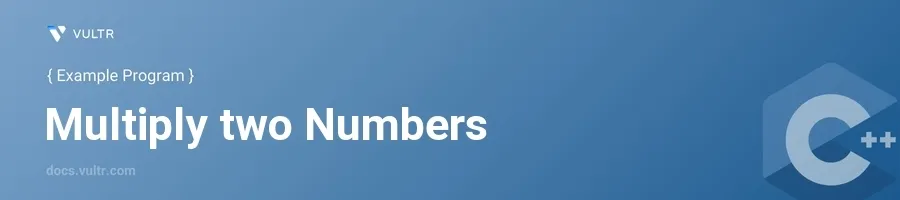
Introduction
Multiplying two numbers is a fundamental operation in programming, often used to demonstrate basic arithmetic capabilities when learning a new language. C++ offers a robust environment for performing mathematical operations efficiently, making it a popular choice for both educational and practical applications.
In this article, you will learn how to use C++ to multiply two numbers. You'll explore various examples showcasing different scenarios where multiplication might be implemented, ranging from simple console inputs to using functions for code reusability.
Basic Multiplication in C++
Direct Multiplication of Two Constants
Declare and initialize two variables to hold the numbers.
Multiply these variables and store the result in a third variable.
Display the multiplication result using the
cout
statement.cpp#include <iostream> using namespace std; int main() { int num1 = 5; int num2 = 3; int product = num1 * num2; cout << "Product: " << product << endl; return 0; }
This example defines two integers, num1 and num2, with values 5 and 3, respectively. The product of these numbers is calculated and then printed.
Multiplying User-provided Values
Prompt the user to enter two numbers.
Use the
cin
function to retrieve the values and store them in variables.Multiply the numbers and output the result.
cpp#include <iostream> using namespace std; int main() { int num1, num2, product; cout << "Enter first number: "; cin >> num1; cout << "Enter second number: "; cin >> num2; product = num1 * num2; cout << "Product: " << product << endl; return 0; }
In this code snippet, the program fetches two integer inputs from the user, multiplies them, and prints the result. It's a practical approach for interactive programs.
Multiplication Using Functions
Function to Multiply Two Numbers
Define a function that takes two numbers as parameters and returns their product.
In your main function, read inputs from the user.
Call the multiplication function with the user inputs and display the result.
cpp#include <iostream> using namespace std; int multiply(int a, int b) { return a * b; } int main() { int num1, num2; cout << "Enter two numbers: "; cin >> num1 >> num2; int product = multiply(num1, num2); cout << "Product: " << product << endl; return 0; }
Here, the function
multiply
is responsible for computing the product of two integers. This method enhances code modularization and reuse.
Using Templates for Different Data Types
Create a template function to multiply numbers of any data type.
Demonstrate the use of the function with various data types.
cpp#include <iostream> using namespace std; template<typename T> T multiply(T a, T b) { return a * b; } int main() { cout << "Int product: " << multiply(3, 4) << endl; cout << "Double product: " << multiply(5.5, 2.2) << endl; return 0; }
The generic function
multiply
utilizes templates to work with any numeric data type. This example shows how to use the function with integers and floating-point numbers, promoting code flexibility and type safety.
Conclusion
In C++, multiplying two numbers is a straightforward task that serves as an excellent introduction to basic programming concepts. Through examples, you've seen how to perform this operation directly, inside functions, and by using templates for type flexibility. Implement these strategies not just for multiplication, but as a stepping stone to handling other mathematical and logical operations in your future C++ projects. The examples provided demonstrate the versatility and potency of C++ in handling arithmetic operations efficiently.
No comments yet.