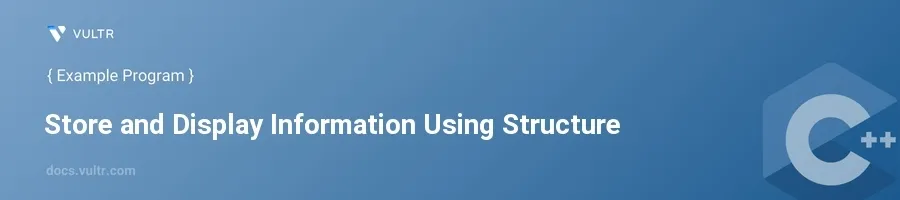
Introduction
In C++, structures are used to group different types of data into a single composite entity. This feature is particularly useful when it comes to storing and managing related data items, such as records or complex data attributes. By using structures, you can create a more organized and modular approach to handling your data in C++ programming.
In this article, you will learn how to define a structure to store information and how to display that information. You'll explore examples that demonstrate the practical use of structures in storing information like student records and employee details, as well as displaying this information correctly.
Understanding Structures in C++
What is a Structure?
- Recognize that a structure in C++ can contain multiple data types.
- Realize that structures help in organizing data efficiently, allowing access through a single entity rather than handling each data item separately.
Declaring a Structure
Define a structure using the
struct
keyword followed by the structure name and a block containing the data members.Declare variables of the structure type just like any other data type.
cppstruct Person { std::string name; int age; float height; };
This code defines a
Person
structure with a name, age, and height, initializing the way to store personal information effectively.
Storing Information Using Structures
Define a Structure for Student Data
Create a structure to store student information including ID, name, and grade.
Initialize structures to add specific student details.
cppstruct Student { int id; std::string name; float grade; };
Initialize Student Data
Define a variable of type
Student
and store information in it.cppStudent student1 = {101, "John Doe", 88.5};
Here,
student1
is initialized with an ID of 101, name "John Doe", and a grade of 88.5.
Displaying Information from Structures
Display Function for Student Structure
Write a function to display information about the student.
Use this function to print the details of a student.
cppvoid displayStudent(Student s) { std::cout << "Student ID: " << s.id << std::endl; std::cout << "Name: " << s.name << std::endl; std::cout << "Grade: " << s.grade << std::endl; }
This function takes a
Student
structure as an argument and prints out the student's ID, name, and grade.
Using the Display Function
Call the display function to show the details of
student1
.cppdisplayStudent(student1);
When this function is called with
student1
, it outputs the student's information stored in the structure.
Struct Arrays for Multiple Records
Understand how to manage multiple data records using an array of structures.
Initialize an array of
Student
type to store multiple records easily.cppStudent classRoom[2] = { {102, "Alice Johnson", 91.2}, {103, "Mark Smith", 84.6} };
This array,
classRoom
, stores information for two students, simplifying data management via structures.Use a loop to display all student records in the array.
cppfor (int i = 0; i < 2; ++i) { displayStudent(classRoom[i]); }
This loop iterates through each element of the
classRoom
array and uses thedisplayStudent
function to print each student's details.
Conclusion
Structures in C++ offer a robust method for managing grouped data. By understanding how to define, initialize, and manipulate structures, you enhance your ability to manage and display complex data efficiently. In scenarios requiring the organization of related data items, such as managing student or employee records, structures prove to be invaluable. Utilize structures in your C++ projects to keep your code organized and maintainable, making data handling tasks straightforward and efficient.
No comments yet.