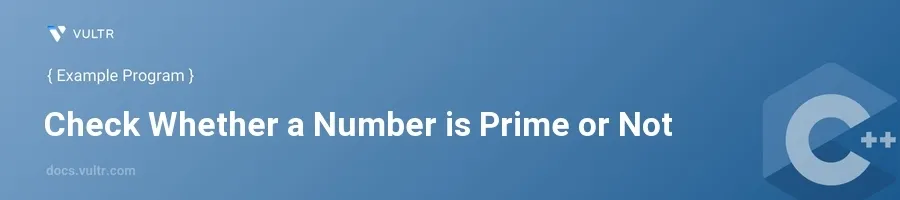
Introduction
Determining whether a number is prime—an integer greater than one that is not a product of two smaller integers—is a fundamental concept in number theory and a common problem in computer programming. It serves as a basis for various cryptographic algorithms, efficient factorization procedures, and even some algorithms in graphic designs and other computational sciences.
In this article, you will learn how to implement a C++ program to check if a number is prime or not. Through detailed examples and explanations, you will discover not just how to execute the function but also optimize it for better performance.
Basic Prime Check in C++
Overview of the Process
- To define whether a number is prime, check if it has no divisors other than 1 and itself.
- Write a function in C++ that takes an integer as input and returns a boolean indicating if the number is prime.
Example: Simple Prime Check
Develop a function named
isPrime()
that iterates through all possible divisors.If it finds a divisor other than 1 or the number itself, return
false
; otherwise, returntrue
.cpp#include <iostream> using namespace std; bool isPrime(int num) { if (num <= 1) return false; for (int i = 2; i < num; i++) { if (num % i == 0) return false; } return true; } int main() { int number; cout << "Enter a number: "; cin >> number; isPrime(number) ? cout << number << " is prime.\n" : cout << number << " is not prime.\n"; return 0; }
This code prompts the user to input a number, checks it using the
isPrime()
function, and prints whether it's prime or not. However, this method is inefficient for large numbers as it iterates through every number less than the given number.
Optimizing the Prime Check
Using Efficient Loop Bounds
Recognize that you only need to check up to the square root of the number because a larger factor of the number would have to multiply with a smaller factor that has already been checked.
Modify
isPrime()
to terminate the loop if it exceeds the square root ofnum
.cpp#include <cmath> using namespace std; bool isPrime(int num) { if (num <= 1) return false; for (int i = 2; i <= sqrt(num); i++) { if (num % i == 0) return false; } return true; }
By checking up to the square root of the number, you significantly reduce the number of iterations needed, especially for large integers. This enhancement makes the function much more efficient.
Checking for Even Numbers
Before diving into the loop, check whether the number is even and greater than two since no even number greater than two is prime.
Exclude all even numbers from the loop by starting at 3 and incrementing by 2 (checking only odd numbers).
cppbool isPrime(int num) { if (num <= 1 || (num % 2 == 0 && num > 2)) return false; for (int i = 3; i <= sqrt(num); i += 2) { if (num % i == 0) return false; } return true; }
This improvement skips half of the unnecessary checks, namely the even numbers greater than two, further reducing the computational load.
Conclusion
The ability to check if a number is prime efficiently is crucial in many areas of computer science and mathematics. By implementing these techniques, you ensure your C++ prime-checking program is both accurate and fast. Use these strategies to optimize algorithms that depend on prime number operations, thereby enhancing your overall program's performance. Each method discussed here—from simple iteration to optimized checks—demonstrates the balance between understanding basic programming concepts and implementing advanced optimization strategies.
No comments yet.