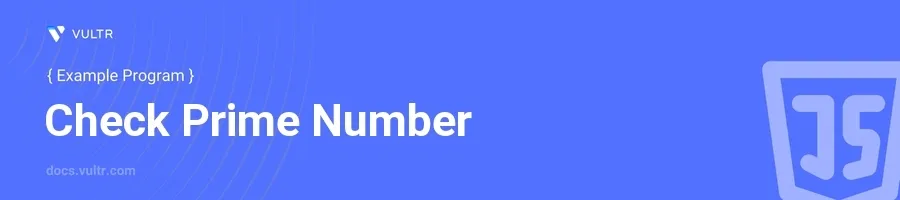
Introduction
A prime number is a natural number greater than 1 that has no positive divisors other than 1 and itself. In other words, it has exactly two distinct positive divisors. Determining whether a number is prime is a common task in various programming challenges and applications, especially in fields like cryptography.
In this article, you will learn how to check if a number is prime using JavaScript. Understanding how to implement this can help you with more complex algorithms and applications where prime numbers play a crucial role. The discussion includes examples that demonstrate different ways to achieve this task effectively.
Basic Approach to Check a Prime Number
Determine Primality
Start by confirming that the number is greater than 1 since any number less than or equal to 1 is not a prime.
Check for factors from 2 up to the square root of the number. If the number is divisible by any of these factors, it is not prime.
javascriptfunction isPrime(num) { if (num <= 1) return false; // numbers less than or equal to 1 are not prime numbers for (let i = 2, sqrt = Math.sqrt(num); i <= sqrt; i++) { if (num % i === 0) return false; } return true; }
In this function,
isPrime
, it begins by checking if the number is less than or equal to 1. It then iterates from 2 to the square root of the number. If the number is divisible by any of these (remainder is 0 when divided), it immediately returnsfalse
. If it completes the loop without finding any factors, it returnstrue
.
Check for Edge Cases
Testing the function with various numbers can ensure its reliability.
javascriptconsole.log(isPrime(11)); // true console.log(isPrime(4)); // false console.log(isPrime(1)); // false console.log(isPrime(17)); // true
Here, the functions output correctly identifies prime numbers and non-prime numbers, including a test for the number 1, which is correctly identified as not prime.
Optimized Approach for Large Numbers
Utilizing Advanced Checks
Implement further optimizations such as checking if the number is even (except for 2, which is prime) and reducing the number of division operations.
javascriptfunction isPrimeOptimized(num) { if (num <= 1) return false; if (num === 2) return true; // 2 is the only even prime number if (num % 2 === 0) return false; // other even numbers are not primes for (let i = 3, sqrt = Math.sqrt(num); i <= sqrt; i += 2) { if (num % i === 0) return false; } return true; }
This optimized version includes checks for even numbers right after checking if the number is less than or equal to 1 and equals 2. It then only checks odd divisors, which halves the iterations needed.
Conclusion
Checking if a number is prime in JavaScript is a straightforward but vital task, especially useful in algorithms and software where prime numbers are an integral part of the logic or problem-solving requirement. By learning how to implement a basic and an optimized approach, you can handle checks efficiently for both small and large numbers. Employ these techniques in relevant projects to elevate the quality and performance of your code.
No comments yet.