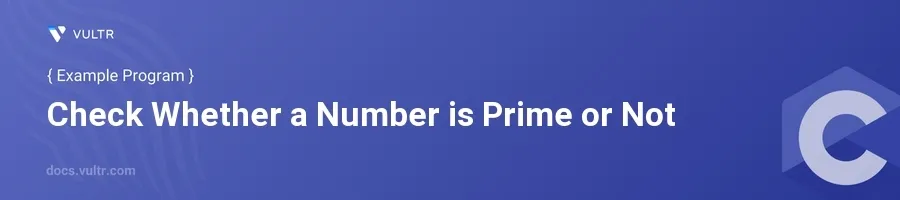
Introduction
Determining whether a number is prime is a common problem in computer science and mathematics. A prime number is a natural number greater than 1 that cannot be formed by multiplying two smaller natural numbers. In the realm of programming, creating a function to check for a prime number is a great way to practice logic building and understand the efficiency of algorithms.
In this article, you will learn how to implement a simple C program to check if a number is prime. Explore practical examples that demonstrate how to use loops and conditionals to solve this classic problem effectively.
Understanding the Prime Number Check
The Prime Check Algorithm
To determine if a number is prime, follow these steps:
Exclude numbers less than 2 as they are not prime.
Check if the number is 2, which is the only even prime number.
For numbers greater than 2, proceed with divisibility checks:
c#include <stdio.h> #include <stdbool.h> bool isPrime(int num) { if (num <= 1) return false; // Step 1: Check for numbers less than 2 if (num == 2) return true; // Step 2: Check the number 2 if (num % 2 == 0) return false; // Step 3: Eliminate even numbers for (int i = 3; i * i <= num; i += 2) { if (num % i == 0) return false; } return true; }
This code defines a function
isPrime()
, which takes an integer and returns a boolean indicating whether the number is prime. It eliminates non-prime numbers through several checks and uses a loop to test divisibility of the number by any odd number up to the square root of the number.
Utilizing the Prime Check Function
Integrate the
isPrime()
function within a main program to test its functionality.Prompt the user to enter a number.
Display the result based on the prime check.
cint main() { int num; printf("Enter a number: "); scanf("%d", &num); if (isPrime(num)) { printf("%d is a prime number.\n", num); } else { printf("%d is not a prime number.\n", num); } return 0; }
In the
main()
function, the program collects a number from the user, runs theisPrime()
check, and prints whether the input is a prime number.
Conclusion
With this C program, you now have a robust tool to check if a number is prime. This example not only helps in understanding basic C syntax but also illustrates the use of functions, loops, and conditional statements. Implement this in various scenarios, such as in educational tools or as part of larger mathematical software, ensuring you efficiently determine prime numbers in your projects.
No comments yet.