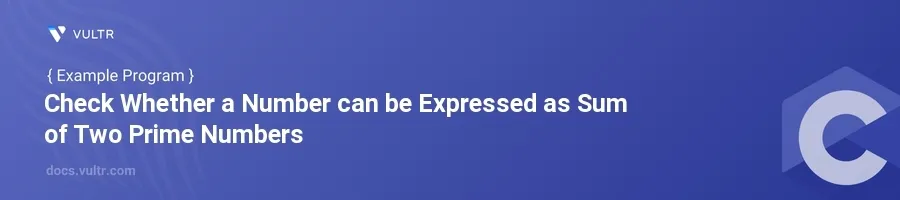
Introduction
Checking whether a number can be expressed as the sum of two prime numbers is a fascinating problem that combines elements of number theory with programming. This challenge not only helps in enhancing algorithm design skills but also deepens understanding of primes, which have significant implications in various fields, particularly cryptography.
In this article, you will learn how to create a C program to determine if a given number can be decomposed into the sum of two prime numbers. Through detailed examples, understand how to implement this check efficiently.
Checking for Prime Numbers
Before solving the main problem, ensure you have a function to check if a number is prime. A prime number is a natural number greater than 1 that has no positive divisors other than 1 and itself.
Function to Check Prime Status
Define a function
isPrime()
which takes an integer and returns1
if the number is prime; otherwise, it returns0
.Iterate from
2
to the square root of the number. If the number is divisible by any of these, it is not prime.c#include <math.h> int isPrime(int num) { if (num <= 1) return 0; for (int i = 2; i <= sqrt(num); i++) { if (num % i == 0) return 0; } return 1; }
This function first handles the case for numbers less than or equal to 1, automatically returning
0
as they are not prime. For other numbers, it checks divisibility up to the square root of the number.
The Program Logic
With the prime checking function ready, proceed to implement the main logic.
Main Program to Check Sum of Two Primes
Accept the target number from the user for which you want to check the condition.
Iterate through numbers and use
isPrime()
to check both the current number and the difference between the target number and the current number.If both numbers are primes, print them; otherwise, continue the loop.
c#include <stdio.h> int main() { int number, i; printf("Enter a number: "); scanf("%d", &number); for (i = 2; i <= number / 2; i++) { if (isPrime(i) && isPrime(number - i)) { printf("%d can be expressed as %d and %d.\n", number, i, number - i); return 0; } } printf("%d cannot be expressed as the sum of two prime numbers.\n", number); return 0; }
This code attempts to decompose the entered number as a sum of any two prime numbers. If it finds such a pair, it outputs the result and terminates. Otherwise, it informs the user that such a decomposition is not possible.
Conclusion
Creating a C program to check if a given number can be expressed as the sum of two prime numbers involves understanding prime number detection and efficiently applying that to solve the main problem. The approach discussed not only helps in solving this specific problem but also lays a foundation for more complex number theory-based programming tasks. With this technique, enhance your programming and problem-solving skills by exploring further variations and optimizations of the solution.
No comments yet.