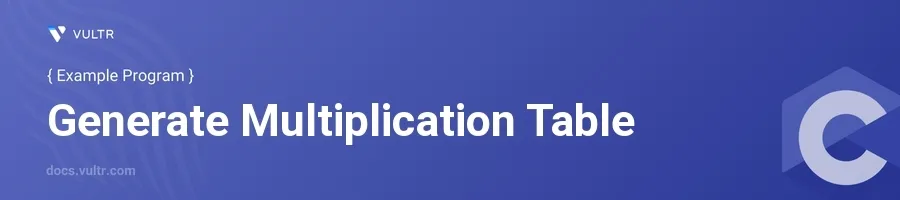
Introduction
A multiplication table is an essential tool used to learn and practice basic multiplication. It shows the products of numbers arranged systematically in rows and columns, which aids in quick reference and calculation. These tables are not only fundamental in educational settings for young students but also useful in various mathematical computations.
In this article, you will learn how to create a C program that generates a multiplication table for any number. You will look at different examples to see how modifications in code can cater to various requirements, such as changing the range of the table or formatting the output for better readability.
Creating a Basic Multiplication Table
Generate a Simple Table for a Given Number
Define the main function and declare necessary variables such as the base number and limit.
Use a
for
loop to iterate through the desired range and print out the multiplication results.c#include <stdio.h> int main() { int number, i; printf("Enter the number to generate the table: "); scanf("%d", &number); for (i = 1; i <= 10; i++) { printf("%d x %d = %d\n", number, i, number * i); } return 0; }
This program prompts the user for a number and generates its multiplication table up to 10. The for
loop iterates from 1 to 10, and within each iteration, the multiplication result is calculated and printed.
Customizing the Range of the Table
Modify the program to allow the user to set their own range for the table.
Adjust the loop to accommodate the dynamic range provided by the user.
c#include <stdio.h> int main() { int number, start, end, i; printf("Enter the number to generate the table: "); scanf("%d", &number); printf("Enter the starting point of the table: "); scanf("%d", &start); printf("Enter the ending point of the table: "); scanf("%d", &end); for (i = start; i <= end; i++) { printf("%d x %d = %d\n", number, i, number * i); } return 0; }
With this modified version, users not only input the base number but also the starting and ending points of the multiplication table. This provides greater flexibility and control over the output.
Enhancing Output Formatting
Improve Table Readability with Aligned Columns
Use formatted output functions to align the multiplication results.
Incorporate tabs or specific spacing to ensure columns are evenly aligned.
c#include <stdio.h> int main() { int number, i; printf("Enter the number to generate the table: "); scanf("%d", &number); printf("\nMultiplication Table of %d:\n\n", number); for (i = 1; i <= 10; i++) { printf("%d x %2d = %2d\n", number, i, number * i); } return 0; }
This code applies formatting with
%2d
to manage the output spacing, ensuring the multiplication table is well-aligned and easy to read.
Conclusion
Building a C program to generate a multiplication table allows you to understand and practice basic C syntax and operations, loops, and formatted output. By adjusting the basic structure, such as modifying the range or improving the output format, you cater to different scenarios and user needs. Implement these techniques to create versatile and user-friendly programs that perform essential mathematical operations efficiently.
No comments yet.