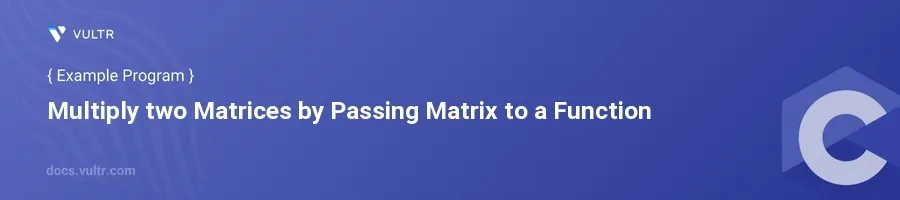
Introduction
Multiplying two matrices in a C program involves a detailed understanding of how matrices can be operated upon programmatically. This task is not only vital in academic courses but also has practical applications in the fields of engineering, computer graphics, and data analysis.
In this article, you will learn how to effectively multiply two matrices by passing them to a function in C. You will explore step-by-step examples that illustrate how to define matrices, pass them to functions, handle matrix multiplication, and return the result.
Defining the Matrix Structure
Create a Structure to Hold Matrix Data
Define a matrix structure which can hold the elements of the matrix and its dimensions.
ctypedef struct { int rows; int cols; int data[10][10]; // Adjust size as needed based on the maximum expected matrix size } Matrix;
This code defines a
Matrix
type with rows and columns, and a two-dimensional array to hold the matrix data.
Function to Multiply Two Matrices
Developing the Matrix Multiplication Function
Write a function that accepts two matrices as parameters and returns the resulting matrix.
cMatrix multiply_matrices(Matrix m1, Matrix m2) { Matrix result; result.rows = m1.rows; result.cols = m2.cols; // Initialize the result matrix with zero for(int i = 0; i < result.rows; i++) { for(int j = 0; j < result.cols; j++) { result.data[i][j] = 0; } } // Matrix multiplication logic for(int i = 0; i < m1.rows; i++) { for(int j = 0; j < m2.cols; j++) { for(int k = 0; k < m1.cols; k++) { result.data[i][j] += m1.data[i][k] * m2.data[k][j]; } } } return result; }
This function first initializes the resulting matrix
result
based on the dimension of matricesm1
andm2
. It then performs matrix multiplication by iterating through rows and columns, updating the result matrix accordingly.
Utilizing the Function in Code
Deployment in a Main Function
Create a
main
function to utilize the multiplication function and display the result.c#include <stdio.h> int main() { Matrix m1 = {2, 3, {{1, 2, 3}, {4, 5, 6}}}; Matrix m2 = {3, 2, {{1, 2}, {3, 4}, {5, 6}}}; Matrix result = multiply_matrices(m1, m2); // Displaying the result printf("Resultant Matrix:\n"); for(int i = 0; i < result.rows; i++) { for(int j = 0; j < result.cols; j++) { printf("%d ", result.data[i][j]); } printf("\n"); } return 0; }
In the
main
function, two matrices,m1
andm2
, are defined and initialized. Themultiply_matrices()
function is called withm1
andm2
as arguments, and the result is stored inresult
. Finally, the resultant matrix is printed to the console.
Conclusion
Multiplying two matrices by passing them to a function in C helps modularize your code and enhances clarity, making it easier to manage and debug. By following the discussed steps and leveraging structures for matrix data, you can handle matrix operations efficiently in your C programs. Whether for academic projects or practical applications, mastering these techniques serves as a strong foundation in programming fundamentals.
No comments yet.