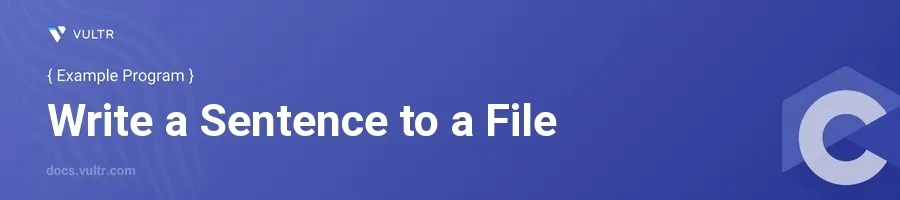
Introduction
In C programming, writing data to files is a fundamental skill that can be applied in many areas, such as logging data, saving output, or working with large datasets that need to persist beyond the execution of the program. File handling in C uses the concepts of file pointers and various functions that allow for both formatted and unformatted writing.
In this article, you will learn how to write a sentence to a file in C using several methods. The article illustrates how to write both using standard library functions like fprintf
and fputs
, ensuring that you understand each method's different applications and benefits.
Writing Using fprintf()
Overview
fprintf()
is similar to the standard printf()
, but instead of writing to the console, it writes to the file. It allows for formatted output, making it highly useful for multiple data types or formatted strings.
Example: Writing a sentence using fprintf()
Open a file in write mode using
fopen()
.Use
fprintf()
to write a formatted string to the file.Close the file using
fclose()
.c#include <stdio.h> int main() { FILE *fp; fp = fopen("example.txt", "w"); if (fp == NULL) { perror("Error opening file"); return(-1); } fprintf(fp, "This is a sentence written to a file using fprintf.\n"); fclose(fp); return 0; }
This code opens
example.txt
in write mode and writes a sentence to it. If the file cannot be opened, it prints an error message.
Writing Using fputs()
Overview
fputs()
writes a string to a file and is easier to use for simple string outputs as it does not support formatting like fprintf()
.
Example: Using fputs() to add a sentence
Open a file in write mode.
Use
fputs()
to write the string.Close the file.
c#include <stdio.h> int main() { FILE *fp; fp = fopen("example.txt", "w"); if (fp == NULL) { perror("Error opening file"); return(-1); } fputs("This sentence was written using fputs.\n", fp); fclose(fp); return 0; }
This code snippet accomplishes a similar task as the previous but using
fputs()
to write to the file. This method is straightforward for writing simple strings.
Conclusion
Writing to files in C is a crucial skill that forms the basis of many more complex file-handling operations. By understanding how to use fprintf()
and fputs()
, you can easily integrate file writing into your C applications, making them capable of handling persistent data and outputting results to files. Each method shown here caters to different needs, offering a flexible approach to file I/O operations in C programs. Apply these techniques to enhance the data management capabilities of your future projects.
No comments yet.