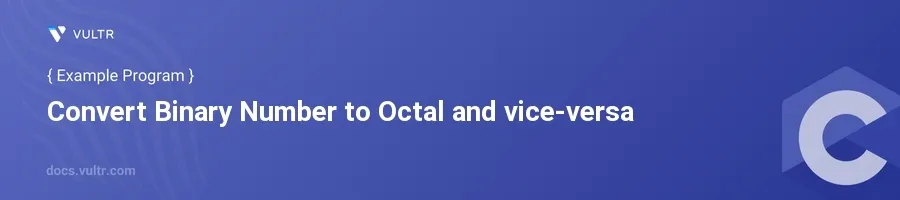
Introduction
Converting numbers between binary and octal systems in programming can be an exciting challenge that tests your understanding of number systems and their manipulations. This process involves translating numbers from a base-2 system (binary) to a base-8 system (octal) and back, which is useful in various computer science applications, such as low-level programming and systems design.
In this article, you will learn how to write C programs to convert a binary number to an octal number and an octal number to a binary number. Follow through clear examples to understand how to implement these conversions efficiently in your programs.
Converting Binary Number to Octal
Understanding the Conversion Process
- Recognize that every group of three binary digits corresponds to one octal digit. This makes the conversion straightforward:
000
to0
001
to1
010
to2
011
to3
100
to4
101
to5
110
to6
111
to7
Example: Binary to Octal Conversion
Define a C function to perform the conversion.
Break the binary number into groups of three from the right and convert each group.
c#include <stdio.h> #include <math.h> int binaryToOctal(long long binary) { int octal = 0, decimal = 0, i = 0; // Converting binary to decimal first while (binary != 0) { decimal += (binary % 10) * pow(2, i); ++i; binary /= 10; } // Converting decimal to octal i = 1; while (decimal != 0) { octal += (decimal % 8) * i; decimal /= 8; i *= 10; } return octal; } int main() { long long binary; printf("Enter a binary number: "); scanf("%lld", &binary); printf("Octal number is: %d", binaryToOctal(binary)); return 0; }
This code includes a function
binaryToOctal()
that converts a binary number to octal by first converting it to decimal and then from decimal to octal. Themain()
function reads a binary input from the user and outputs the converted octal value.
Converting Octal Number to Binary
Understanding the Conversion Process
- Realize that each octal digit directly translates to three binary digits.
Example: Octal to Binary Conversion
Create a function to map each octal digit to its corresponding binary form.
Process each digit individually, starting from the most significant digit (leftmost).
c#include <stdio.h> #include <string.h> void octalToBinary(int octal) { int decimal = 0, i = 0; long long binary = 0; // Converting octal to decimal while (octal != 0) { decimal += (octal % 10) * pow(8, i); ++i; octal /= 10; } // Converting decimal to binary i = 1; while (decimal != 0) { binary += (decimal % 2) * i; decimal /= 2; i *= 10; } printf("Binary number is: %lld", binary); } int main() { int octal; printf("Enter an octal number: "); scanf("%d", &octal); octalToBinary(octal); return 0; }
Here, the function
octalToBinary()
first converts an octal number to a decimal, and then from decimal to binary. Themain()
function facilitates user input for the octal number and displays the binary output.
Conclusion
By exploring the conversion mechanisms between binary and octal number systems, you enhance your programming skills in C and gain a deeper understanding of data representation in computing. The examples provided demonstrate direct methods to convert numbers in binary to octal and vice versa, using intermediate decimal conversions. Utilize these strategies in your projects to effectively manage different numeric forms in low-level programming tasks.
No comments yet.