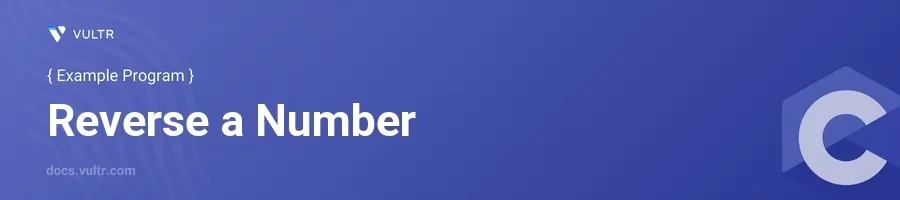
Introduction
Reversing a number is a common task in programming that tests your ability to manipulate data structures and understand algorithmic logic. In C programming, this concept involves converting the number into its opposite sequence, which can serve different purposes, such as creating palindromic numbers or simplifying mathematical computations.
In this article, you will learn how to reverse a number using C programming through practical examples. Discover how to handle both integer reversals and consider the implications of negative numbers in such operations.
Basic Number Reversal
Reversing a Positive Integer
Start by initializing and reading the input number.
Use a loop to reverse the digits of the number.
Output the reversed number.
c#include <stdio.h> int main() { int num, reversed = 0; printf("Enter an integer: "); scanf("%d", &num); while(num != 0) { int digit = num % 10; reversed = reversed * 10 + digit; num /= 10; } printf("Reversed Number: %d\n", reversed); return 0; }
This code takes an integer input, extracts each digit, and reconstructs the number in reverse order. The key operation
reversed = reversed * 10 + digit
shifts the current digits left and adds the new digit to the right.
Reversal of a Negative Integer
Confirm the number is negative and convert it to a positive before reversing.
Follow similar steps as reversing a positive number.
Convert the result back to negative to maintain the original sign.
c#include <stdio.h> int main() { int num, reversed = 0; printf("Enter an integer: "); scanf("%d", &num); int isNegative = num < 0 ? 1 : 0; if (isNegative) num = -num; while(num != 0) { int digit = num % 10; reversed = reversed * 10 + digit; num /= 10; } if (isNegative) reversed = -reversed; printf("Reversed Number: %d\n", reversed); return 0; }
This approach adjusts for negative numbers by temporally converting the number to positive, applying the reversal, then switching the result back to negative.
Edge Cases Consideration
Dealing with Leading Zeros
While a C program generally ignores leading zeros in integer calculations, understanding how they affect data when presented in different contexts (like strings or numbers) is crucial.
Zero and Single-Digit Numbers
Acknowledge that for numbers between -9 and 9, the reversal is the number itself.
Implement a condition to handle this specific scenario efficiently.
cif (num >= -9 && num <= 9) { printf("Reversed Number: %d\n", num); return 0; }
This snippet directly outputs the number if it lies within the specified range, skipping the unnecessary reversal logic.
Conclusion
Reverse a number in C by following the methods described, adapting as necessary for variables like number sign and magnitude. By mastering these techniques, you enhance your ability to solve related problems, such as creating palindromic numbers or preparing for interviews that test your algorithmic thinking capabilities. Utilize these strategies to ensure your solutions are both efficient and robust.
No comments yet.