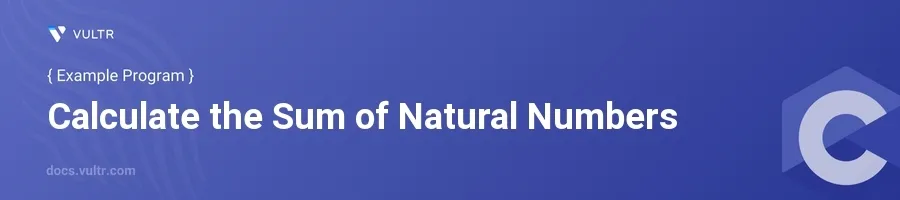
Introduction
Calculating the sum of natural numbers is a fundamental problem in computer programming that demonstrates the use of loops and basic arithmetic operations. Such programs are often used as introductory tasks to help new programmers understand the flow of control in a language like C.
In this article, you will learn how to write a C program to calculate the sum of natural numbers through various examples. Explore different ways to implement this, enhancing your understanding of loops and conditional statements in C.
Example 1: Using a For Loop
Calculating Sum Using a For Loop
Start by defining the main function where you'll implement the sum calculation.
Use a for loop to iterate from 1 up to and including a given number
n
.In each iteration, add the current number to a sum variable initialized at zero before the loop starts.
c#include <stdio.h> int main() { int n, sum = 0; printf("Enter a positive integer: "); scanf("%d", &n); for (int i = 1; i <= n; i++) { sum += i; } printf("Sum = %d", sum); return 0; }
This C program prompts the user to enter a positive integer, then calculates the sum of all natural numbers up to that integer using a for loop. The sum is accumulated in the variable
sum
, which is outputted at the end of the loop.
Example 2: Using a While Loop
Implementing Sum Calculation with a While Loop
Declare and initialize variables for the number, sum, and an iterator.
Use a while loop that runs until the iterator exceeds the number.
Inside the loop, add the value of the iterator to the sum and then increment the iterator.
c#include <stdio.h> int main() { int n, sum = 0, i = 1; printf("Enter a positive integer: "); scanf("%d", &n); while (i <= n) { sum += i; i++; } printf("Sum = %d", sum); return 0; }
The snippet above achieves the same result as the previous example but uses a while loop. This method provides a clear demonstration of how a while loop can be used to iterate based on a condition that changes within the loop itself.
Example 3: Using Mathematical Formula
Direct Calculation Using the Formula
Understand that the sum of the first
n
natural numbers can be directly calculated using the formula: ( \text{Sum} = \frac{n(n + 1)}{2} ).Implement this formula in a program to calculate the sum without using loops.
c#include <stdio.h> int main() { int n, sum; printf("Enter a positive integer: "); scanf("%d", &n); sum = n * (n + 1) / 2; printf("Sum = %d", sum); return 0; }
This method is the most efficient because it eliminates the need for loops and computes the sum in constant time. It's a direct application of the formula for the sum of the first
n
natural numbers.
Conclusion
Creating a C program to calculate the sum of natural numbers can be approached in several ways, each providing unique insights into the use of loops, conditionals, and mathematical formulas. From using iterative structures like for and while loops to applying a direct formula, these methods offer varied levels of complexity and performance. Use these techniques depending on the situation and your need for efficiency or simplicity in explaining the concepts to beginners.
No comments yet.