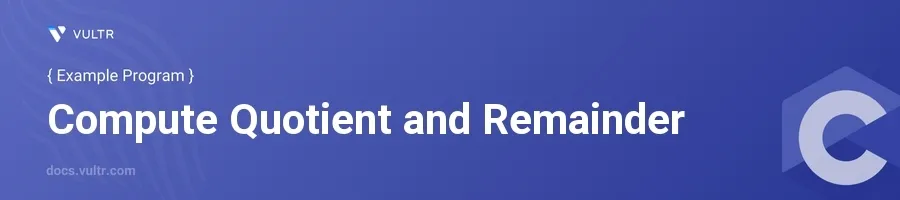
Introduction
In C programming, computing the quotient and remainder is a fundamental task often encountered when performing arithmetic operations. These operations are essential for various applications, including mathematical computations, algorithms, and even in systems programming where resource division is necessary.
In this article, you will learn how to compute the quotient and remainder of two numbers using C. You’ll explore several examples that demonstrate simple arithmetic operations and how to implement them to achieve desired results.
Computing Quotient and Remainder in C
Using the Division and Modulus Operators
Computing the quotient and remainder involves two primary operators in C: the division operator (/
) and the modulus operator (%
). The division operator gives the quotient, while the modulus operator yields the remainder.
Define two integer variables for the dividend and divisor.
Use the division operator for computing the quotient.
Use the modulus operator for computing the remainder.
Print the results using
printf
.c#include <stdio.h> int main() { int dividend = 29; int divisor = 5; int quotient = dividend / divisor; int remainder = dividend % divisor; printf("Quotient: %d\n", quotient); printf("Remainder: %d\n", remainder); return 0; }
In this code snippet,
dividend
is divided bydivisor
to find the quotient and remainder.29 / 5
gives a quotient of5
and a remainder of4
. The results are then printed out.
Handling Negative Numbers
When working with negative numbers, the behavior of the modulus operator in C can vary depending on the compiler and platform. It's important to test the behavior in the specific development environment.
Change the values of
dividend
and/ordivisor
to negative and note the outcome.Print the results for comparison.
c#include <stdio.h> int main() { int dividend = -29; int divisor = 5; int quotient = dividend / divisor; int remainder = dividend % divisor; printf("Quotient: %d\n", quotient); printf("Remainder: %d\n", remainder); return 0; }
Here, with
dividend
as-29
anddivisor
as5
, the result typically would be a quotient of-5
and a remainder of-4
, but this output can vary.
Conclusion
Computing the quotient and remainder using the division and modulus operators in C is straightforward, and applying these operators helps solve many practical programming problems. Remember to consider how negative numbers are handled in your specific C environment, as this can affect the output of modulus operations. Through the examples provided, tailor your approach to computing with these operators effectively within your projects.
No comments yet.