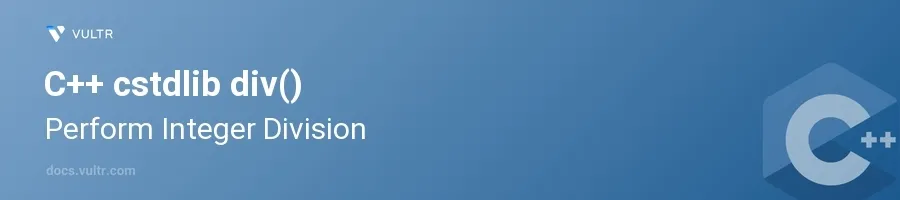
Introduction
The div()
function from the C++ cstdlib
library provides a structured way to perform integer division, returning both the quotient and the remainder in a single operation. This simplifies tasks in many programming scenarios, especially where both division results are required simultaneously without performing separate operations.
In this article, you will learn how to use the div()
function for efficient integer division. Explore how to integrate it into your C++ projects to handle calculations that require the quotient and remainder from dividing two integers.
Understanding div() Function
Syntax and Return Type
Import the necessary header file.
c++#include <cstdlib>
Understand the syntax of the
div()
function.c++div_t div(int numer, int denom);
numer
: The numerator in the division.denom
: The denominator in the division.- Returns a structure
div_t
that contains two integers:quot
(the quotient) andrem
(the remainder).
How to Use div() in Programs
Basic Division Operation
Include the
cstdlib
library.Define the numerator and denominator.
Call the
div()
function and store the result.Print the quotient and remainder.
c++#include <iostream> #include <cstdlib> int main() { int numerator = 20; int denominator = 3; div_t result = div(numerator, denominator); std::cout << "Quotient: " << result.quot << std::endl; std::cout << "Remainder: " << result.rem << std::endl; return 0; }
This example divides 20 by 3, resulting in a quotient of 6 and a remainder of 2. The
div()
function encapsulates both results in theresult
variable.
Handling Negative Values
Be aware that
div()
correctly handles negative values.c++#include <iostream> #include <cstdlib> int main() { int numerator = -20; int denominator = 3; div_t result = div(numerator, denominator); std::cout << "Quotient: " << result.quot << std::endl; std::cout << "Remainder: " << result.rem << std::endl; return 0; }
If either the numerator or denominator is negative, the
div()
function handles this gracefully, giving the appropriate mathematical results with proper signs.
Common Pitfalls and Tips
- Ensure the denominator is not zero, as dividing by zero will cause runtime errors.
- Understand that
div()
works specifically with integers. For floating-point division, other functions or operators must be used.
Conclusion
The div()
function in C++ from the cstdlib
library is a practical tool for performing integer division, yielding both the quotient and remainder efficiently. By using div()
, you streamline your code, avoiding separate calculations for the quotient and remainder, which not only simplifies your code but also improves its execution speed. Practice integrating this function into various mathematical operations in your C++ projects to appreciate its utility fully.
No comments yet.