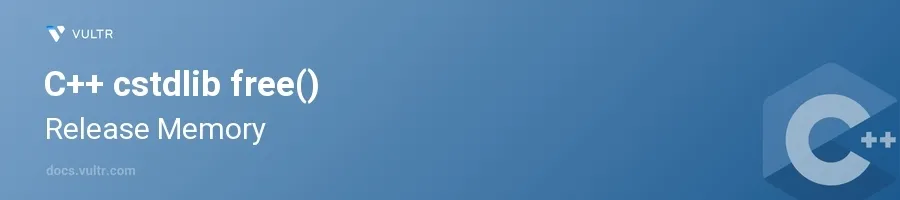
Introduction
The free()
function from the C++ cstdlib
library is essential for managing dynamic memory allocation. It is used to release memory that was previously allocated with malloc()
, calloc()
, or realloc()
. Ensuring that unused memory is properly freed is crucial for both performance and effective resource management in C++ applications.
In this article, you will learn how to correctly utilize the free()
function to release memory in C++. This includes understanding its importance, pinpointing when to use it, dealing with multiple memory blocks, and managing possible issues related to memory management.
Understanding the free() Function
Correct Usage of free()
Allocate memory using
malloc()
or a similar function.Perform necessary operations with the allocated memory.
Release the memory using
free()
.cppint* ptr = (int*) malloc(10 * sizeof(int)); // Allocating memory for 10 integers free(ptr); // Freeing the memory
In this example, memory is first allocated to an integer pointer and then released using
free()
. It is crucial to pass the exact pointer returned bymalloc()
or similar functions tofree()
; otherwise, undefined behavior may occur.
Importance of free()
- Understand that failure to free allocated memory leads to memory leaks.
- Realize that long-running programs can consume all available memory if they do not free unused memory.
When to Use free()
- Always use
free()
after the memory allocated bymalloc()
,calloc()
, orrealloc()
is no longer needed. - Use
free()
immediately before a pointer goes out of scope if no further use for the allocated memory exists.
Managing Memory with free()
Dealing with Multiple Memory Blocks
Each allocated block must be individually freed.
Forgetting to free any of the blocks results in partial memory leaks.
cppint* block1 = (int*) malloc(10 * sizeof(int)); int* block2 = (int*) malloc(20 * sizeof(int)); // Operations on block1 and block2 free(block1); free(block2);
Here, two blocks of memory are allocated and later freed. Remembering to free each separately is essential to avoid memory leaks.
Common Mistakes and Issues
Avoid calling
free()
on a pointer that has already been freed (double free
error).Ensure that you do not use a memory after it has been freed (
use after free
error).cppint* p = (int*) malloc(sizeof(int)); *p = 10; // Using memory free(p); // p should not be used from here onwards
Avoid using
p
after it has been freed to prevent runtime errors.
Conclusion
Using the free()
function in C++ is a best practice for any developer dealing with dynamic memory allocation. Knowing when and how to use this function ensures that your applications run efficiently without wasting memory resources. Employ it effectively to manage memory in various C++ projects, thus preventing possible memory-related issues that can degrade your application's performance and reliability. By adhering to the techniques outlined, you maintain robust and efficient memory management in your software development endeavors.
No comments yet.