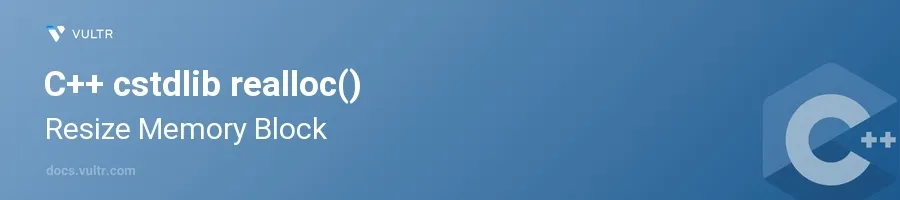
Introduction
The realloc()
function in C++ is a vital component of the cstdlib
library, allowing for the dynamic resizing of memory blocks initially allocated with malloc()
or calloc()
. This function is commonly used to modify the size of an existing memory block, thus making it adaptable to changing data requirements within an application.
In this article, you will learn how to effectively utilize the realloc()
function to resize memory blocks. You’ll explore different scenarios that might necessitate the use of realloc()
, how to handle potential errors, and important considerations to ensure that your program remains robust and efficient.
Understanding realloc()
Basic Usage of realloc()
Allocate memory using
malloc()
orcalloc()
.Check if the initial memory allocation is successful.
Use
realloc()
to resize the memory block.cpp#include <cstdlib> #include <iostream> int main() { // Initial allocation int* ptr = (int*)malloc(5 * sizeof(int)); // Check for allocation failure if (!ptr) { std::cerr << "Initial memory allocation failed.\n"; return 1; } // Resize memory block ptr = (int*)realloc(ptr, 10 * sizeof(int)); // Check if reallocation was successful if (!ptr) { std::cerr << "Memory reallocation failed.\n"; free(ptr); return 1; } std::cout << "Memory reallocated successfully.\n"; // Clean up free(ptr); return 0; }
In this example, memory is first allocated for five integers and then resized to hold ten integers. The program checks for allocation failures before and after resizing to ensure reliability.
Handling realloc() Errors
Always assign the result of
realloc()
to a temporary pointer variable.Free the original block if
realloc()
fails to prevent memory leaks.cppint* tempPtr = (int*)realloc(originalPtr, new_size * sizeof(int)); if (!tempPtr) { std::cerr << "Failed to reallocate memory.\n"; free(originalPtr); // Prevent memory leak } else { originalPtr = tempPtr; // Use newly allocated memory }
This code snippet demonstrates using a temporary pointer to handle potential
realloc()
failures. It keeps the program safe from memory leaks by properly managing memory.
Best Practices with realloc()
Avoid using
realloc()
on pointers not allocated withmalloc()
orcalloc()
.Always check the result of
realloc()
before using the memory to avoid undefined behaviors.Remember that
realloc(NULL, size)
is equivalent tomalloc(size)
.Free the memory block if it is no longer needed to prevent memory leaks.
By following these best practices, you ensure the safe and effective use of memory in your applications, minimizing errors and maximizing resource efficiency.
Conclusion
The realloc()
function in the C++ cstdlib
library is essential for managing dynamic memory allocations in applications that require flexible handling of data. By understanding how to use realloc()
correctly, you can ensure that your programs handle memory efficiently and are robust against common errors such as memory leaks. Keep in mind the various scenarios and best practices discussed to make optimal use of realloc()
in your projects.
No comments yet.