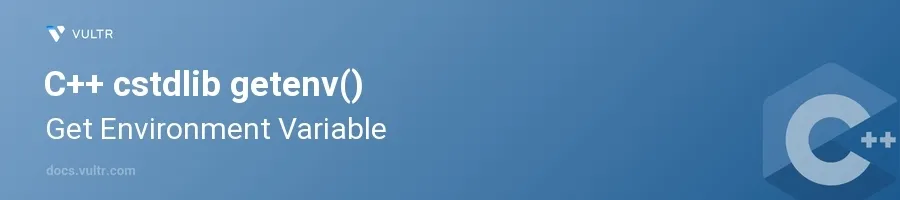
Introduction
The getenv()
function, which is part of the C++ standard library cstdlib
, provides a straightforward way to access environment variables from within a C++ application. Environment variables are dynamic values which affect the processes or programs on a computer. They can store information such as drive, path, or file locations, and are often used for configuration settings and to control the behavior of various processes.
In this article, you will learn how to effectively utilize the getenv()
function to fetch environment variable values. Discover practical examples to understand how to integrate this method into your C++ applications effectively.
Using getenv() to Access Environment Variables
Retrieve a Specific Environment Variable
Include the
cstdlib
library, wheregetenv()
is defined.Use
getenv()
to fetch the value of a specific environment variable.cpp#include <cstdlib> #include <iostream> int main() { const char* value = std::getenv("PATH"); if (value != nullptr) { std::cout << "PATH: " << value << std::endl; } else { std::cout << "PATH environment variable is not set." << std::endl; } return 0; }
This code attempts to retrieve the value of the
PATH
environment variable. It checks whether the returned pointer isnullptr
to determine if the environment variable is set. If the variable exists, it prints the value; otherwise, it notifies that the variable is not set.
Handling Non-Existent Environment Variables
Understand that
getenv()
returns a pointer to achar
, which isnullptr
if the environment variable does not exist.Implement error checking to ensure your program handles this situation gracefully.
cpp#include <cstdlib> #include <iostream> int main() { const char* value = std::getenv("NON_EXISTENT_VAR"); if (value != nullptr) { std::cout << "Variable exists: " << value << std::endl; } else { std::cout << "Variable does not exist." << std::endl; } return 0; }
In this example, the code checks for an environment variable named
NON_EXISTENT_VAR
. Since this variable likely does not exist,getenv()
returnsnullptr
, and the program prints a message stating the variable does not exist.
Conclusion
The getenv()
function in C++ provides a simple and efficient way to access environment variables. It is especially useful in situations where your application’s behavior needs to adapt based on the runtime environment without hard-coding specific values. By incorporating the techniques discussed, ensure your code effectively handles the retrieval of environment variable values, enhancing both functionality and reliability of your C++ applications.
No comments yet.