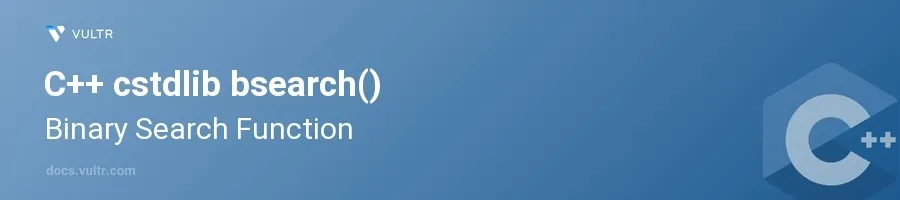
Introduction
The bsearch()
function in C++ resides within the <cstdlib>
library and serves as a tool for performing binary searches on sorted arrays. This function is pivotal when searching for specific elements quickly within a large dataset, as binary search methodology substantially decreases the number of comparisons needed compared to linear search techniques.
In this article, you will learn how to effectively leverage the bsearch()
function to find elements in sorted arrays. Explore how to set up the function, use a comparator for different data types, and understand the conditions under which this function operates most effectively.
Understanding bsearch()
Setup Requirements for bsearch()
Include the
<cstdlib>
header in your C++ program.Ensure the array is sorted before applying
bsearch()
since the function assumes a sorted array for its operation.Understand the function signature of
bsearch()
:cppvoid* bsearch(const void* key, const void* base, size_t num, size_t size, int (*compar)(const void*, const void*));
Here's a breakdown of the parameters:
key
: pointer to the element you are searching for.base
: pointer to the first element in the array.num
: total number of elements in the array.size
: size of each element in bytes.compar
: pointer to a comparison function that determines the sorting order.
Writing a Comparator Function
Define a comparator function that matches the
bsearch()
requirements.Ensure the comparator function returns an integer less than, equal to, or greater than zero, corresponding to whether the first argument is considered less than, equal to, or greater than the second argument.
cppint compareInts(const void* a, const void* b) { int int_a = *(int*)a; int int_b = *(int*)b; if (int_a < int_b) return -1; else if (int_a > int_b) return 1; else return 0; }
This comparator can be used for an array of integers where the comparison is based on integer values.
Implementing bsearch() in Practical Scenarios
Searching in an Integer Array
Create and sort an array of integers.
Use
bsearch()
with the integer array and the custom comparator.cppint arr[] = {1, 2, 4, 8, 16, 32}; int key = 8; int* result = (int*) bsearch(&key, arr, 6, sizeof(int), compareInts); if (result) { std::cout << "Found " << key << " at index " << (result - arr) << std::endl; } else { std::cout << "Element not found." << std::endl; }
This code snippet uses
bsearch()
to find the number8
in a sorted array. The function returns a pointer to the element if found, null otherwise.
Adaptation for Other Data Types
Modify or write another comparator suitable for the data type, e.g., for strings.
Adjust the array type and key type used in the
bsearch()
function call.cppint compareStrings(const void* a, const void* b) { return strcmp(*(const char**)a, *(const char**)b); }
Use a similar structure for calling
bsearch()
with the appropriate types and comparator.
Conclusion
The bsearch()
function in C++ provides a powerful way to perform fast searches on sorted arrays, significantly reducing the search time over linear methods. Successful implementation requires understanding of its parameters, creation of a suitable comparator, and ensuring that arrays are appropriately sorted before search begins. Adapt the methods and comparators shown to cater to various data types and scenarios, thus optimizing code efficiency and capability. Remember to handle the edge cases where elements may not be found, ensuring your program's robustness and reliability.
No comments yet.