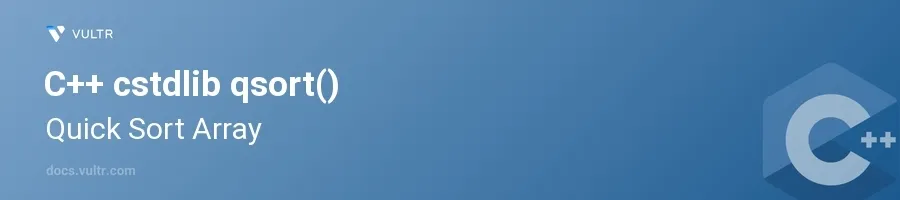
Introduction
The qsort()
function, part of C++'s <cstdlib>
library, serves as a generic quicksort implementation for sorting arrays. Due to its flexibility, it can sort arrays of any data type with a comparison function tailored to the specific type. Whether dealing with integers, strings, or custom structs, qsort()
equips developers with the power to sort based on their specific needs and conditions.
In this article, you will learn how to effectively utilize the qsort()
function to sort arrays in C++. You'll discover how to define comparison functions for different data types and see example implementations that demonstrate the sorting of integers and custom structs.
Basics of Using qsort()
The qsort()
function requires four arguments:
- A pointer to the array to be sorted.
- The number of elements in the array.
- The size of each element.
- A comparison function that determines the order of elements.
Basic Syntax of qsort()
Here's how you generally set up a call to qsort()
:
#include <cstdlib>
void qsort(void *base, size_t num, size_t size, int (*compar)(const void*, const void*));
base
: Pointer to the array to be sorted.num
: Number of elements in the array.size
: Size of each element in the array.compar
: Pointer to the comparison function.
Example: Sorting an Array of Integers
Define a comparison function for integers.
cppint compare_ints(const void* a, const void* b) { const int* arg1 = (const int*)a; const int* arg2 = (const int*)b; if (*arg1 < *arg2) return -1; if (*arg1 > *arg2) return 1; return 0; }
This function takes two pointers as arguments, dereferences them, and returns:
-1
if the first integer is less than the second.1
if the first integer is greater than the second.0
if they are equal.
Sort an array using
qsort()
.cppint main() { int arr[] = {10, 5, 15, 3, 12}; int n = sizeof(arr) / sizeof(arr[0]); qsort(arr, n, sizeof(int), compare_ints); for (int i = 0; i < n; i++) printf("%d ", arr[i]); return 0; }
This code sorts the array
arr
of integers in ascending order. The sorted array is then printed in the main function.
Sorting Custom Data Types
qsort()
is not limited to primitive data types. It can also sort arrays of custom structs by using an appropriate comparison function.
Example: Sorting an Array of a Custom Struct
Define the struct and a comparison function.
cppstruct Person { char name[100]; int age; }; int compare_persons(const void* a, const void* b) { const Person* person1 = (const Person*) a; const Person* person2 = (const Person*) b; return person1->age - person2->age; }
Sort an array of
Person
structs usingqsort()
.cppint main() { Person people[] = {{"Alice", 30}, {"Bob", 25}, {"Charlie", 35}}; int n = sizeof(people) / sizeof(people[0]); qsort(people, n, sizeof(Person), compare_persons); for (int i = 0; i < n; i++) printf("%s : %d\n", people[i].name, people[i].age); return 0; }
This implementation sorts the array
people
based on their age in ascending order. The sorted data is then displayed.
Conclusion
The qsort()
function from C++'s <cstdlib>
library is a versatile and powerful tool that can sort arrays of any type. By creating a customized comparison function, you unlock the potential to sort based on various criteria. Whether working with simple integers or complex user-defined types, qsort()
provides a robust solution for organizing data efficiently and effectively. Leverage this function in your projects to enhance data handling and improve performance.
No comments yet.