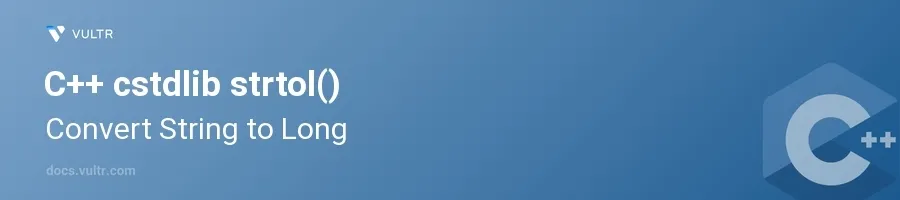
Introduction
The strtol()
function in C++ is a part of the cstdlib library, designed to convert strings to a long integer (long). This utility is especially valuable when dealing with user input or parsing strings to extract numbers that are integral to the application's logic, such as configuration settings, numerical data processing, and more.
In this article, you will learn how to effectively use the strtol()
function in various programming situations. Discover how this function helps in type conversion, handles invalid input, and works around certain edge cases to robustly integrate string-to-long conversion in your C++ applications.
Understanding strtol()
Basic Usage of strtol()
Include the cstdlib header to have access to
strtol()
.Prepare a string that represents a long integer.
Use
strtol()
to convert the string.cpp#include <cstdlib> char str[] = "1024"; char *pEnd; long int li = strtol(str, &pEnd, 10);
Here,
strtol()
converts the stringstr
into a long integer. The third argument10
represents the base, which in this case is decimal.pEnd
will point to the part of the string following the numeric value, which in this example should point to the string's null terminator as there are no characters after the number.
Handling Invalid Input
Check for numbers with non-numeric suffixes or prefixes.
Apply
strtol()
and examine the pointer to where the numeric conversion stopped.cppchar input[] = "1234abc"; long int number = strtol(input, &pEnd, 10); if (*pEnd != '\0') { std::cout << "Conversion stopped at: " << pEnd << std::endl; }
Here, you attempt to convert
input
usingstrtol()
. The function will convert the1234
part and then stop atabc
because it's not a valid numeric character, which you can then handle accordingly.
Working With Different Bases
Understand that
strtol()
can convert strings in various bases (from 2 to 36).Convert a hexadecimal string using
strtol()
.cppchar hexStr[] = "1A3F"; long int hexNumber = strtol(hexStr, nullptr, 16); std::cout << "Decimal: " << hexNumber << std::endl;
This code snippet takes a hexadecimal number represented as a string (
"1A3F"
) and converts it into a long integer using base 16. If the conversion is successful, it will print the decimal equivalent of the hexadecimal number.
Using strtol() Effectively
Error Handling
Use the
errno
from<cerrno>
to detect overflows and underflows.Test
strtol()
with a larger-than-long value.cpp#include <cerrno> errno = 0; // reset errno to 0 before conversion attempt char largeNum[] = "999999999999999999999999999999"; long int large = strtol(largeNum, &pEnd, 10); if (errno == ERANGE) { std::cout << "Overflow or underflow occurred.\n"; }
In this section, if an overflow occurs due to the magnitude of the number in
largeNum
,strtol()
setserrno
toERANGE
. By checkingerrno
, you can effectively manage such errors and take necessary actions like logging the error or prompting the user for a different input.
Conclusion
The strtol()
function in C++ is an invaluable tool for converting strings to long integers in a variety of contexts. By understanding and handling special cases such as different numeric bases and invalid inputs, you enhance the robustness of your applications. Whether dealing with user inputs in a UI application or reading configuration files in a system program, strtol()
provides the functionality needed to correctly interpret and use numeric values embedded in strings. Implement these strategies to ensure your code remains reliable and effective in handling string-to-number conversions.
No comments yet.