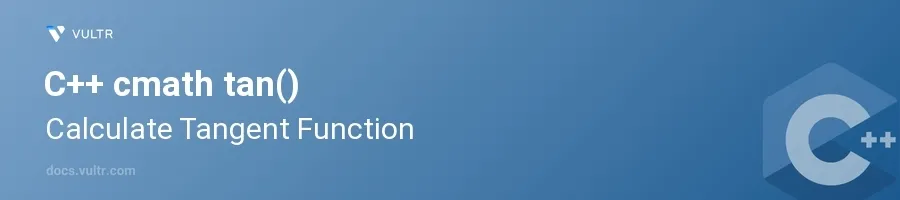
Introduction
The tan()
function in C++ is part of the cmath library, used to calculate the tangent of an angle given in radians. This mathematical function is widely used in fields such as engineering, physics, and computer graphics for performing trigonometric calculations precisely.
In this article, you will learn how to effectively use the tan()
function in C++. The examples provided will demonstrate how to calculate the tangent for both specific angles and dynamically during runtime, along with handling specific mathematical considerations such as angles that yield undefined tangent values.
Utilizing tan() in C++
Calculating Tangent of a Specific Angle
Include the cmath library to access the
tan()
function.Define an angle in radians.
Calculate the tangent of the angle using
tan()
.cpp#include <iostream> #include <cmath> int main() { double angle = M_PI / 4; // 45 degrees double tangent = std::tan(angle); std::cout << "The tangent of 45 degrees is: " << tangent << std::endl; return 0; }
This code calculates the tangent of 45 degrees (π/4 radians). The output should approximate 1, as the tangent of 45 degrees equals 1.
Handling Angles with Undefined Tangent
Understand that the tangent function has undefined values at odd multiples of π/2 (90 degrees, 270 degrees, etc.).
Check if the angle is an odd multiple of π/2 before calling
tan()
.Provide an appropriate response or handling mechanism for these cases.
cpp#include <iostream> #include <cmath> #include <limits> int main() { double angle = M_PI / 2; // 90 degrees if (fmod(angle, M_PI / 2) == 0 && (int)(angle / (M_PI / 2)) % 2 != 0) { std::cout << "The tangent of 90 degrees is undefined." << std::endl; } else { double tangent = std::tan(angle); std::cout << "The tangent of the angle is: " << tangent << std::endl; } return 0; }
In this example, the code checks if
angle
is an odd multiple of π/2, where the tangent function is classically undefined. If it is, it outputs a message indicating so; otherwise, it calculates the tangent.
Dynamic Tangent Calculation
Prompt the user to input an angle.
Calculate and display the tangent of the entered angle.
cpp#include <iostream> #include <cmath> int main() { double angle; std::cout << "Enter an angle in radians: "; std::cin >> angle; double tangent = std::tan(angle); std::cout << "The tangent of " << angle << " radians is: " << tangent << std::endl; return 0; }
This program takes an angle in radians from the user and calculates its tangent, which is then displayed. This approach allows for dynamic calculation based on user input.
Conclusion
Using the tan()
function from the cmath library in C++ enables precise calculations of the tangent of angles specified in radians. Whether integrating this function into physics simulations, engineering calculations, or real-time applications, adhering to the examples and considerations outlined ensures that your trigonometric computations are both accurate and robust. Employ these strategies to enhance the mathematical component of your C++ programs efficiently.
No comments yet.