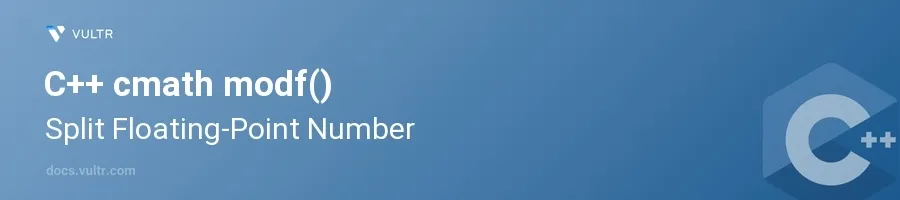
Introduction
The modf()
function in C++ is a useful utility provided in the <cmath>
library. It splits a floating-point number into its integer and fractional parts, both of which retain the sign of the input value. This ability makes it especially useful in graphics, simulation, and real-time processing where the separation of these components is frequently required.
In this article, you will learn how to leverage the modf()
function from the C++ cmath
library. Discover how to split a floating-point number into its integral and fractional components and utilize these parts in various programming contexts.
Using C++ cmath modf()
Splitting a Floating-point Number
The modf()
function takes two parameters: the floating-point number you want to split and a pointer to a double where it will store the integer part of the split. The function returns the fractional part of the number.
Include the C++ cmath library.
Declare a variable to hold your floating-point number and another to hold the integer part.
Use the
modf()
function to split the number.cpp#include <iostream> #include <cmath> int main() { double number = 9.1567; double intPart; double fracPart = modf(number, &intPart); std::cout << "Integer part: " << intPart << std::endl; std::cout << "Fractional part: " << fracPart << std::endl; return 0; }
Here,
number
is split intointPart
andfracPart
. TheintPart
stores the integer component9
, and the function returns the fractional component0.1567
which is captured infracPart
.
Handling Negative Values
Recognize that modf()
preserves the sign of the decimal number, so the integer and fractional parts will have the same sign as the input number.
Use a negative floating-point number.
Apply the
modf()
function similarly to before.cpp#include <cmath> #include <iostream> int main() { double number = -23.87; double intPart; double fracPart = modf(number, &intPart); std::cout << "Integer part: " << intPart << std::endl; std::cout << "Fractional part: " << fracPart << std::endl; return 0; }
In this example, the input number is
-23.87
. Themodf()
function splits it intointPart
with-23
and returns afracPart
of-0.87
.
Conclusion
The modf()
function in C++ is a versatile tool for extracting the integer and fractional parts of a floating-point number, while maintaining the sign of the original input. Its utility spans multiple programming scenarios, particularly those involving numeric computations and graphical transformations. By grasping how to implement and use this function, optimize data processing tasks and increase the precision of your computational results. Use the examples and explanations provided to integrate the modf()
function into your C++ projects, enhancing your code’s capability to handle floating-point arithmetic effectively.
No comments yet.