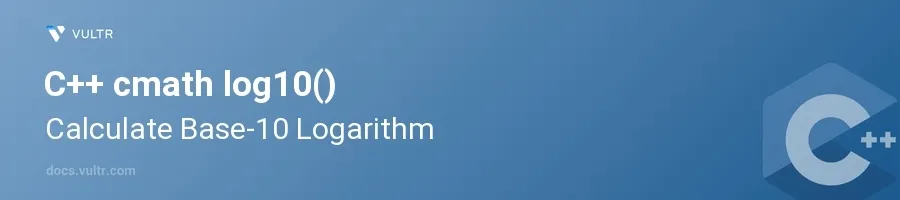
Introduction
The log10()
function (aka log base 10) in C++ is a part of the cmath
library and is used to compute the base-10 logarithm of the given number. This function is crucial for calculations involving exponential scaling, scientific data processing, and engineering applications where logarithmic values are essential for formulas and analyses.
In this article, you will learn how to effectively use the log10()
function when working with different numerical data types in C++. Explore common scenarios in C++ programming where log base 10 calculation is needed, ensuring you apprehend the scope and utility of this function.
Basic Usage of log10() Function in C++
Calculating Base 10 Logarithm of a Positive Number
Include the
cmath
library.Initialize a positive number.
Use
log10()
to calculate the logarithm.c++#include <cmath> #include <iostream> double number = 1000.0; double result = log10(number); std::cout << "Log10 of " << number << " is " << result << std::endl;
This code calculates the base-10 logarithm of
1000
, which equals3
. The result is displayed using standard output.
Handling Errors When Using log10() in C++
Recognize that
log10()
requires the argument to be greater than 0.Illustrate an error case where the input is non-positive.
c++#include <cmath> #include <iostream> #include <cerrno> #include <cstring> double number = 0.0; double result = log10(number); if (errno != 0) { std::cerr << "Error: " << std::strerror(errno) << std::endl; } else if (result == -HUGE_VAL) { std::cerr << "Log10 of zero is negative infinity" << std::endl; }
Using a number like
0
will seterrno
and return negative infinity, indicating the mathematical error, as logarithm of0
is undefined.
Interfacing log10() Function with Other Data Types in C++
Calculating Base-10 Logarithm of an Integer in C++
Note that
log10()
can also handle integer types directly.Convert the result back to an integer if necessary.
c++#include <cmath> #include <iostream> int number = 1000; double result = log10(number); std::cout << "Log10 of " << number << " is " << static_cast<int>(result) << std::endl;
Even though
number
is an integer,log10()
computes its logarithm correctly, demonstrating the function’s versatility with different data types.
Working with Negative Numbers through Complex Logarithm
Understand that directly using
log10()
on negative numbers is invalid.Use
std::complex
to handle negative inputs when calculating logarithms.c++#include <cmath> #include <iostream> #include <complex> std::complex<double> number(-1000.0, 0.0); std::complex<double> result = log10(number); std::cout << "Log10 of " << number << " is " << result << std::endl;
For negative or complex numbers, you use
std::complex
to avoid domain errors, and the result is presented as a complex number.
Conclusion
The log10()
function in C++ provides a straightforward way to calculate base-10 logarithms, which are widely used in scientific computations, data analysis, and engineering applications. By handling edge cases such as zero and negative inputs, and understanding how log10()
interacts with different numeric types—including integers and floating-point values—you can write more reliable and accurate code. Integrating log10()
into your C++ programs not only supports exponential and logarithmic scaling but also enhances your software's ability to perform complex mathematical operations with confidence.
No comments yet.