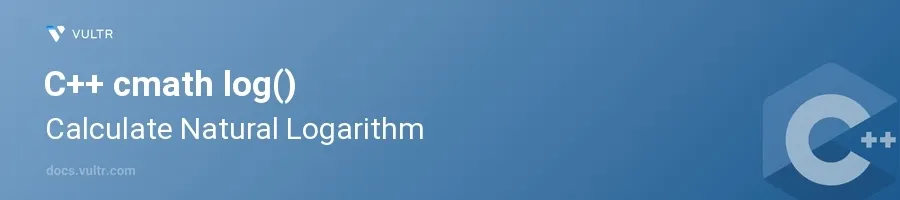
Introduction
The log()
function in the C++ cmath
library computes the natural logarithm (base e
) of a given input. Natural logarithm calculations are critical in various scientific, engineering, and financial computations where exponential growth or decay is involved. This function is essential for analyzing trends and changes that follow a logarithmic scale.
In this article, you will learn how to use the log()
function effectively in C++. You will explore how to handle different types of inputs, deal with potential errors, and see practical examples that help illustrate its use in real-world applications.
Applying the log() Function
Basic Usage of log()
Include the
<cmath>
header in your C++ program to access thelog()
function.Call the
log()
function with a numeric argument.cpp#include <iostream> #include <cmath> int main() { double value = 2.718; double result = log(value); std::cout << "Natural logarithm of " << value << " is " << result; return 0; }
This code computes the natural logarithm of approximately
e
(2.718), which should ideally return a value close to 1. Thelog()
function is part of thecmath
library and calculates the natural logarithm.
Working with Special Values
Understand how
log()
behaves with different types of special input values, such as negatives and zero.Implement checks before using
log()
to avoid domain errors.cpp#include <iostream> #include <cmath> #include <limits> int main() { double values[] = {0.0, -1.0, 1.0}; for(double value : values) { if(value <= 0) { std::cout << "Log not defined for " << value << std::endl; } else { double result = log(value); std::cout << "Natural logarithm of " << value << " is " << result << std::endl; } } return 0; }
Here, the code iterates through an array containing
0.0
,-1.0
, and1.0
. Thelog()
function is not defined for non-positive values, and usinglog()
with such values without checks could lead to undefined behavior or domain errors.
Usage in Complex Calculations
Incorporate the
log()
function into more complex mathematical formulas where the natural logarithm is a part.Use
log()
to solve real-world problems involving exponential growth or decay, such as calculating compound interest or half-life periods.cpp#include <iostream> #include <cmath> int main() { double rate = 0.05; // 5% growth per year double time = 20; // 20 years double initialAmount = 1000; // initial amount of $1000 // Calculate final amount after 20 years of continuous growth double finalAmount = initialAmount * exp(rate * time); std::cout << "Final amount after 20 years: $" << finalAmount << std::endl; // Calculate time required to double the initial amount double doubleTime = log(2) / rate; std::cout << "Time required to double the initial amount: " << doubleTime << " years" << std::endl; return 0; }
In this example,
exp()
is used together withlog()
to model continuous growth and calculate the time required to double an investment at a constant annual growth rate. Thelog()
function helps convert the goal of doubling the investment into the time needed at a given rate.
Conclusion
The log()
function from the C++ cmath
library is indispensable for computations involving natural logarithms. Mastering its usage, along with understanding the considerations of different input values, enriches the capability to tackle various computational problems in science, finance, and engineering. By integrating the knowledge gained here, boost your efficiency in solving complex calculations that involve logarithmic functions.
No comments yet.