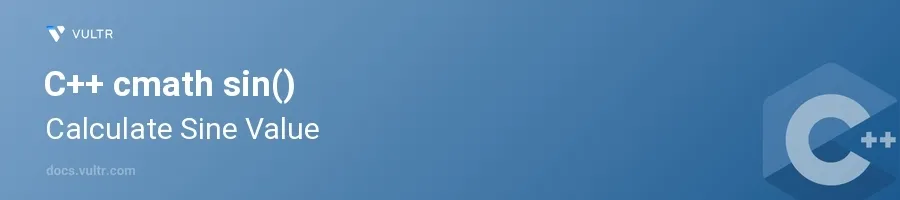
Introduction
The sin()
function from the C++ <cmath>
library is essential for computing the sine of an angle, which plays a crucial role in fields ranging from engineering to game development. This function takes an angle in radians and returns its sine, a fundamental trigonometric calculation.
In this article, you will learn how to leverage the sin()
function to calculate sine values for different angles. Gain insights into working with radians, handling trigonometric computations in C++, and applying these concepts in practical scenarios.
Calculating Sine Values in C++
Basic Usage of sin()
Include the
<cmath>
library.Declare a variable for the angle in radians.
Call the
sin()
function with the angle.cpp#include <cmath> #include <iostream> int main() { // Angle in radians double radians = M_PI / 2; // 90 degrees double sineValue = sin(radians); std::cout << "Sine of 90 degrees: " << sineValue << std::endl; return 0; }
This code calculates the sine of 90 degrees, which is
1
. It demonstrates thatM_PI
represents π in C++ and helps convert degrees to radians.
Converting Degrees to Radians
Understand that angles need to be in radians for
sin()
.Convert degrees to radians using the formula ( \text{Radians} = \text{Degrees} \times \frac{\pi}{180} ).
cpp#include <cmath> #include <iostream> int main() { double degrees = 30; double radians = degrees * M_PI / 180; double sineValue = sin(radians); std::cout << "Sine of 30 degrees: " << sineValue << std::endl; return 0; }
This snippet converts 30 degrees into radians before using the
sin()
function. The result corresponds to the sine of 30 degrees, approximately0.5
.
Using sin() with Negative Angles
Use negative values for angles to find sine of angles in the negative direction.
Utilize the same conversion and computation pattern.
cpp#include <cmath> #include <iostream> int main() { double degrees = -45; double radians = degrees * M_PI / 180; double sineValue = sin(radians); std::cout << "Sine of -45 degrees: " << sineValue << std::endl; return 0; }
Calculate the sine of -45 degrees (or 315 degrees when considered positive in standard position), producing
-0.7071067811865475
.
Conclusion
The sin()
function in C++ is a powerful tool for performing trigonometric calculations, crucial for anything from physics simulations to animation. By understanding how to convert degrees to radians and apply the sin()
function, you enhance your ability to implement mathematical functions accurately within your applications. Experiment with these techniques to handle various angle calculations efficiently and accurately.
No comments yet.