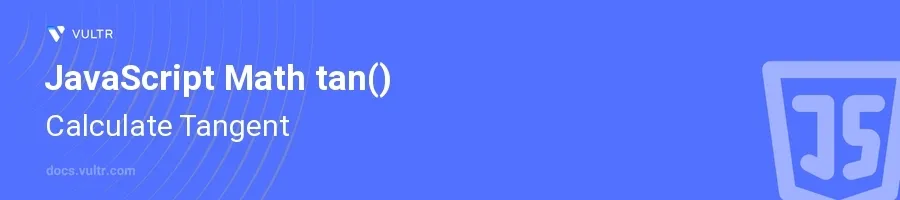
Introduction
The Math.tan()
function in JavaScript is a built-in method that calculates the tangent of a number. This trigonometric function works with angles expressed in radians and is part of the larger Math
object, which provides various mathematical constants and functions. As a fundamental component in geometry, physics, and engineering computations where angle measurements are crucial, understanding how to use Math.tan()
enhances the capabilities of any JavaScript developer working with numeric computations.
In this article, you will learn how to effectively use the Math.tan()
function in various scenarios. Explore practical examples to calculate the tangent of different angles and understand the output based on the input supplied in radians.
Basic Usage of Math.tan()
Calculate Tangent of an Angle
Convert the angle from degrees to radians because
Math.tan()
requires the input in radians.Use
Math.tan()
to calculate the tangent.javascriptconst degrees = 45; const radians = degrees * (Math.PI / 180); const tangent = Math.tan(radians); console.log(tangent);
In this example, the angle is 45 degrees, which is converted to radians. The tangent of 45 degrees is calculated, which theoretically should be 1 in a perfect scenario. The output reflects this expected value.
Understanding Outputs for Various Angles
Calculate tangents for a series of angles to see how
Math.tan()
behaves with different inputs.Execute the calculations and print the results.
javascriptconst angles = [0, 30, 45, 60, 90]; angles.forEach((degree) => { const radian = degree * (Math.PI / 180); const tangent = Math.tan(radian); console.log(`Tangent of ${degree} degrees is: ${tangent}`); });
This code snippet processes multiple angles, displaying their tangent values. Notably, as the angle approaches 90 degrees, the tangent value rapidly increases, illustrating the properties of the tangent function near π/2 radians (90 degrees).
Handling Special Cases
Tangent of 90 Degrees
Recognize that the tangent of 90 degrees (or π/2 radians) should technically be undefined because it approaches infinity.
Apply
Math.tan()
to see JavaScript's handling of this mathematical scenario.javascriptconst tangent90 = Math.tan(Math.PI / 2); console.log(tangent90);
When you calculate the tangent of 90 degrees using JavaScript, the output is a very large number, indicating an approach toward infinity (
Infinity
). This result is significant for error handling in practical applications.
Negative Angles and Their Tangents
Realize that tangents of negative angles return negative values, as tangent is an odd function.
Calculate and compare the tangent of a positive angle and its negative counterpart.
javascriptconst posAngle = 45; // positive 45 degrees const negAngle = -45; // negative 45 degrees const tangentPos = Math.tan(posAngle * (Math.PI / 180)); const tangentNeg = Math.tan(negAngle * (Math.PI / 180)); console.log(`Tangent of +45 degrees: ${tangentPos}`); console.log(`Tangent of -45 degrees: ${tangentNeg}`);
In this comparison, notice how the tangent of -45 degrees is the negative of the tangent of 45 degrees. This is due to the symmetric properties of the tangent function around the origin.
Conclusion
The Math.tan()
function in JavaScript offers a straightforward method to compute the tangent of angles in radians. Employ this function to handle geometry-based calculations, perform simulations, or manage any scenario that incorporates angle calculations. The examples and scenarios provided guide you on how to converse angles from degrees to radians and explore the function behavior under various conditions. Master these techniques to make your numerical computation tasks easier and more efficient.
No comments yet.