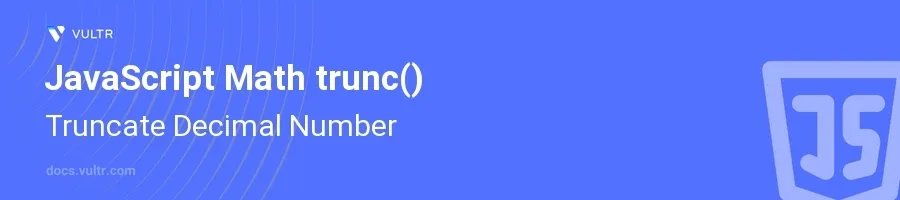
Introduction
The Math.trunc()
function in JavaScript truncates a decimal number to its integer part, disregarding the fractional digits. This method is particularly useful when you need to remove the decimal part of a number without rounding it in any direction. Unlike other rounding methods like Math.floor()
and Math.ceil()
, Math.trunc()
simply cuts off the decimal part, making it straightforward and predictable for handling floating-point numbers.
In this article, you will learn how to effectively use the Math.trunc()
function in various scenarios. Explore the basic usage of this function and delve into more advanced examples that deal with positive and negative numbers, as well as practical applications in mathematical programming.
Basic Usage of Math.trunc()
Truncating a Positive Decimal Number
Define a positive decimal number.
Apply the
Math.trunc()
function to remove the decimal part.javascriptlet positiveNum = 8.93; let truncatedPositive = Math.trunc(positiveNum); console.log(truncatedPositive);
This code snippet will output
8
, showing thatMath.trunc()
has successfully removed the decimal part of the number.
Truncating a Negative Decimal Number
Start with a negative decimal number.
Use the
Math.trunc()
function to truncate the number.javascriptlet negativeNum = -4.27; let truncatedNegative = Math.trunc(negativeNum); console.log(truncatedNegative);
In this example, the output will be
-4
. TheMath.trunc()
function effectively truncates the number towards zero, which means it always removes the decimal part without rounding.
Advanced Applications of Math.trunc()
Using Math.trunc() for Input Validation
Consider inputs where users can enter floating-point numbers but you require whole integers.
Utilize
Math.trunc()
to sanitize these inputs.javascriptfunction validateInput(input) { return Math.trunc(input); } console.log(validateInput(3.78)); // Outputs: 3 console.log(validateInput(-1.99)); // Outputs: -1
Here,
Math.trunc()
ensures that any floating-point number provided by the user is converted to an integer by removing the fractional part. This technique is helpful in scenarios where decimal numbers can cause errors or unexpected behavior.
Handling Data with Decimal Points
Gather data that often contains decimal points, like measurement or currency data.
Employ
Math.trunc()
to standardize these data points to integers for certain types of analyses or displays.javascriptlet prices = [19.99, 34.48, 20.00, 15.67]; let truncatedPrices = prices.map(price => Math.trunc(price)); console.log(truncatedPrices); // Outputs: [19, 34, 20, 15]
This method is useful for preparing raw data for summary statistics, display in user interfaces, or further processing where non-integer values could complicate the logic.
Conclusion
The Math.trunc()
function in JavaScript offers a straightforward way to truncate numbers to their integer components by simply removing the fractional part. It's useful for preparing and sanitizing data, ensuring consistent mathematical calculations, and simplifying the handling of numeric inputs across various programming scenarios. By employing the techniques discussed, you streamline your number handling processes, making your JavaScript code more robust and dependable.
No comments yet.