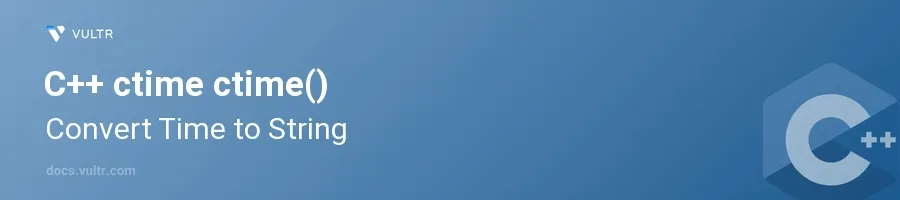
Introduction
The C++ ctime()
function from the <ctime>
header file provides a convenient way to convert a time value to a human-readable string. This function forms part of the C standard library and serves as a straightforward approach for displaying time information formatted as a string, which can be particularly useful for logging, reports, or any user-facing outputs that require time stamping.
In this article, you will learn how to use the ctime()
function effectively in your C++ applications. Discover how to integrate this function to convert time values to strings, handle possible issues, and utilize this capability for enhancing data readability and logging.
Basics of ctime()
Understanding ctime() Syntax and Usage
Include the
ctime
library in your C++ program.Use
ctime()
by passing it a pointer to a time value, typically obtained from time-related functions liketime()
.cpp#include <iostream> #include <ctime> int main() { time_t current_time = time(nullptr); // Get the current time char* readable_time = ctime(¤t_time); // Convert to readable format std::cout << "Current Time: " << readable_time << std::endl; return 0; }
This code snippet first includes the necessary headers
<iostream>
for output and<ctime>
for time-related functions. It fetches the current time usingtime(nullptr)
and then converts this time to a human-readable format usingctime()
.
Handling Null Pointers and Errors
Ensure the pointer passed to
ctime()
is not null to avoid undefined behavior.Check the output of
ctime()
as it returns a null pointer if the time cannot be converted.Ensure that the
time_t
value is valid and the system time settings do not cause the time value to roll over into an undefined state.
Advanced Uses of ctime()
Formatting and Locale Settings
Be aware that the output of
ctime()
is affected by the system’s locale settings.To customize time formatting, consider using
strftime()
for more control over the output format.cpp#include <iostream> #include <ctime> int main() { char buf[80]; time_t current_time = time(nullptr); struct tm *timeinfo = localtime(¤t_time); strftime(buf, sizeof(buf), "%A, %B %d, %Y %H:%M:%S", timeinfo); std::cout << "Formatted Time: " << buf << std::endl; return 0; }
This example demonstrates using
strftime()
to achieve a customizable string format for time, which provides more flexibility compared toctime()
.
Conclusion
The ctime()
function in C++ is a handy tool for converting time values to a broadly understandable string format. While its use is simple and direct, be mindful of system locale settings and potential for null values which can affect its output. For applications requiring more customized time formats, consider leveraging strftime()
, which offers greater control. With these insights, you can enhance data readability and improve time-related implementations in your software projects.
No comments yet.