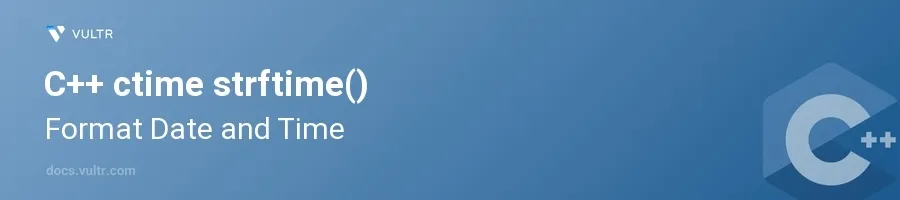
Introduction
The strftime()
function in C++ is a powerful utility found in the <ctime>
library, used for formatting date and time into a string according to specified formats. It provides flexibility in how date and time are represented in applications, making it essential for logging, reporting, and user-interface design where precise or human-readable date and time representations are necessary.
In this article, you will learn how to use the strftime()
function effectively in various date and time formatting scenarios. Explore the function's format specifiers and understand how to employ them in real-world C++ applications to meet your formatting needs.
Understanding strftime() Basics
Basic Usage of strftime()
Include the necessary header file.
Initiate a
tm
structure to hold the date and time.Use
strftime()
to format the date and time as desired.cpp#include <ctime> #include <iostream> int main() { char formatted[100]; time_t t = time(nullptr); tm* timePtr = localtime(&t); strftime(formatted, sizeof(formatted), "Today is %A, %B %d, %Y", timePtr); std::cout << formatted << std::endl; return 0; }
Here,
strftime()
is used to format the current date and time into a more human-readable string according to the format "Today is Day, Month Date, Year".
Customizing Time Display
Explore additional format specifiers to include time specifics.
Format the string to include hours, minutes, and seconds.
cppstrftime(formatted, sizeof(formatted), "Current time: %H:%M:%S", timePtr); std::cout << formatted << std::endl;
This additional code formats the current time in a 24-hour clock format showing hours, minutes, and seconds.
Advanced strftime() Formatting
Using Locale-specific Formats
Understand the influence of locale settings on date and time formatting.
Set a specific locale and format the date and time accordingly.
cpp#include <locale> std::locale::global(std::locale("en_US.UTF-8")); strftime(formatted, sizeof(formatted), "%c", timePtr); std::cout << formatted << std::endl;
By setting the locale to "en_US.UTF-8",
strftime()
formats the date and time string according to the US cultural norms.
Handling Non-standard Format Characters
Realize that some non-standard extensions may vary by the implementation.
Experiment with compiler-specific extensions or standards.
cppstrftime(formatted, sizeof(formatted), "Week of the year: %V", timePtr); std::cout << formatted << std::endl;
Using
%V
to attempt fetching the ISO 8601 week number of the year, which is supported by some C++ library implementations.
Conclusion
The strftime()
function in C++ from the <ctime>
library is indispensable for formatting date and time. It allows the construction of custom date-time strings easily adaptable to various local and international formats. Employ this function to enhance how you handle temporal data in your C++ projects, ensuring you can display dates and times in the most appropriate formats for your application's needs. By mastering strftime()
, you maintain control over temporal data presentation and make your applications more intuitive and user-friendly.
No comments yet.