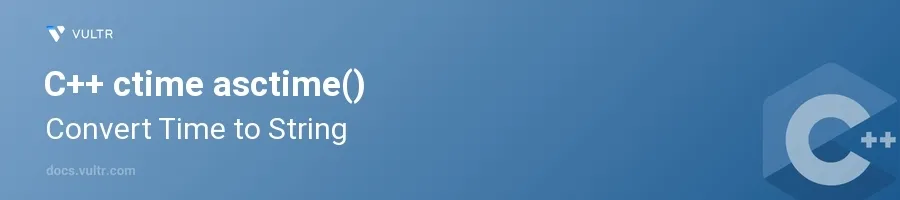
Introduction
The asctime()
function in C++ is a part of the C standard library and provides a convenient way to convert time data into a human-readable string. It is particularly useful in applications where you need to display or log the date and time in a standard format. This function takes a pointer to a tm
structure as an argument and returns a string representing the local time formatted as Www Mmm dd hh:mm:ss yyyy
.
In this article, you will learn how to use the asctime()
function to convert tm
struct time data to a readable string format. You'll explore how to set up a tm
structure, use asctime()
to convert this time, and then handle the output properly for display or further processing.
Working with asctime()
Setup a tm Structure
Before utilizing
asctime()
, you need to create and populate atm
structure with the current time.Use
time()
to get the current time andlocaltime()
to convert this time into atm
structure.cpp#include <ctime> #include <iostream> int main() { time_t now = time(0); tm *ltm = localtime(&now);
Here,
time(0)
fetches the current time, andlocaltime()
converts thistime_t
value into atm
structure localized to the system's time zone.
Convert Time Using asctime()
With the
tm
structure ready, pass it toasctime()
to convert it to a readable string.Print the resulting string to see the formatted time.
cppchar* time_str = asctime(ltm); std::cout << "Current time: " << time_str << std::endl; return 0; }
The
asctime(ltm)
function takes the local time information stored inltm
and returns a pointer to a string that represents this time in a human-readable format. The output might look likeThu Aug 23 14:55:02 2023
.
Considerations When Using asctime()
Recognize that
asctime()
returns a pointer to a statically allocated string.Understand that subsequent calls to
asctime()
can overwrite this string.Avoid issues with overwriting by copying the string if you need to use the result multiple times or across different parts of your program. Use string copy functions appropriately to manage this.
Conclusion
The asctime()
function in C++ is an effective way to convert time from a tm
structure to a readable string format. It simplifies the process of formatting and displaying dates and times in applications. By following the steps outlined, you can easily implement time display features in your C++ programs. Remember to handle the output of asctime()
cautiously, especially in multi-threaded contexts or scenarios requiring multiple time conversions, to maintain data integrity.
No comments yet.