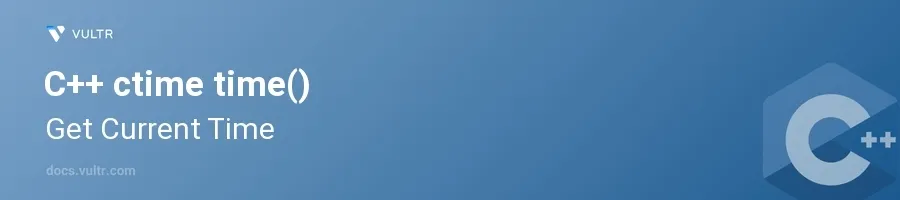
Introduction
The time()
function in the C++ ctime
library is commonly used to retrieve the current system time. This function provides a simple way of accessing the Unix timestamp, which represents the number of seconds elapsed since January 1, 1970. It's an essential part of many applications, such as logging events, time-stamping actions, or measuring durations within your C++ programs.
In this article, you will learn how to use the time()
function to acquire the current time in your C++ programs. Explore practical examples demonstrating how to utilize this function for various purposes, like generating timestamps and comparing time intervals.
Retrieving Current Time Using time()
Acquiring the Current Timestamp
Include the necessary library.
Declare a variable of type
time_t
to store the time.Call
time()
and pass the address of the variable created.cpp#include <ctime> #include <iostream> time_t now; time(&now); std::cout << "Current time: " << now << " seconds since the Unix epoch" << std::endl;
This code snippet initializes
now
with the current Unix time, i.e., the number of seconds since January 1, 1970. The result is displayed to the console.
Formatting the Current Time
Understand that
time_t
is typically used with otherctime
functions for formatting.Convert
time_t
to a human-readable format.cpp#include <ctime> #include <iostream> time_t now; time(&now); char* dt = ctime(&now); std::cout << "Current date and time: " << dt << std::endl;
In this example,
ctime
converts thetime_t
objectnow
into a human-readable string that represents the local time.
Calculating Time Intervals
Measuring a Duration
Record the start time.
Execute the operations whose duration needs measurement.
Record the end time and compute the difference.
cpp#include <ctime> #include <iostream> time_t start_time; time(&start_time); // Assume some operations here time_t end_time; time(&end_time); double duration = difftime(end_time, start_time); std::cout << "Operation took " << duration << " seconds." << std::endl;
This snippet calculates the duration of operations by taking the difference between
end_time
andstart_time
using thedifftime
function.
Conclusion
The time()
function in C++'s ctime
module is a robust tool for obtaining and manipulating time values in your applications. Whether you need to display the current time, timestamp events, or measure the duration of operations, time()
proves highly effective. Implement these techniques to enhance the functionality and usability of your C++ projects by incorporating precise timing features.
No comments yet.