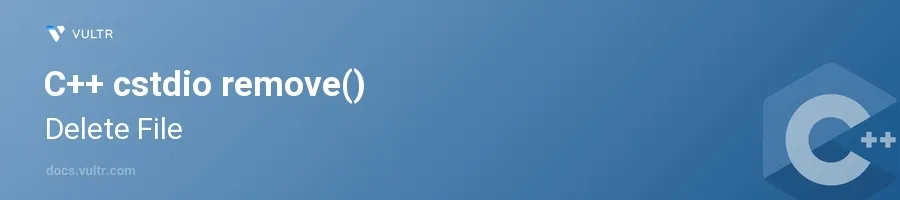
Introduction
The remove()
function in C++ is a standard library function used to delete files (and sometimes directories, depending on the operating system and filesystem). Specifically, it's part of the <cstdio>
library, which provides functionalities for C-style file input and output operations. This function is essential for file system management in C++ programs, giving programmers straightforward means to handle file deletions directly through their code.
In this article, you will learn how to leverage the remove()
function to delete files in your C++ applications. Explore practical examples that demonstrate the use of this function to handle common file management tasks securely and efficiently.
Understanding the remove() Function
Function Syntax and Parameters
Familiarize yourself with the basic syntax of the
remove()
function:c++int remove(const char *filename);
filename
: A C-style string representing the path to the file you want to delete.- Returns an integer:
0
if the file is successfully deleted, or a non-zero value if an error occurs.
Basic Example of Deleting a File
Create a simple program to delete a specified file.
c++#include <cstdio> int main() { const char* filename = "example.txt"; if (remove(filename) == 0) { printf("File deleted successfully.\n"); } else { perror("Error deleting file"); } return 0; }
This example attempts to delete a file named
"example.txt"
. The program checks the return value ofremove()
. If it's0
, the file was successfully deleted; otherwise, it prints an error message.
Handling Errors with remove()
Understanding Common Errors
- Know the common reasons why
remove()
might fail:- The file does not exist.
- The program does not have the necessary permissions to delete the file.
- The file is currently open or being used by another process.
Improved Error Handling
Enhance the file deletion program with better error handling to understand specific failure cases.
c++#include <cstdio> int main() { const char* filename = "example.txt"; if (remove(filename) == 0) { printf("File deleted successfully.\n"); } else { perror("Failed to delete file"); } return 0; }
In this version,
perror()
is used to provide a message that gives more insight into why the deletion may have failed, based on system error codes.
Use Cases and Practical Applications
Automating File Cleanup in Applications
- Integrate file deletion logic into applications to manage temporary files or clean up old data automatically.
Conditional File Deletion
Implement logic to delete files only under certain conditions, such as files beyond a specific age or those matching certain patterns.
c++#include <cstdio> #include <filesystem> namespace fs = std::filesystem; int main() { fs::path path_to_check = "old_logs"; for (const auto& entry : fs::directory_iterator(path_to_check)) { if (fs::is_regular_file(entry) && entry.last_write_time() < fs::file_time_type::clock::now() - std::chrono::days(30)) { remove(entry.path().c_str()); } } return 0; }
This snippet checks a directory and deletes files that have not been modified in the last 30 days. Note that C++17 or later is required for
std::filesystem
.
Conclusion
The remove()
function in C++ offers a straightforward approach to handle file deletions within programs. By understanding its structure and return behavior, you can effectively integrate file deletion capabilities into your C++ applications, automate clean-up tasks, and handle various file management scenarios robustly. Embrace these techniques to ensure your applications can manage their file storage efficiently.
No comments yet.