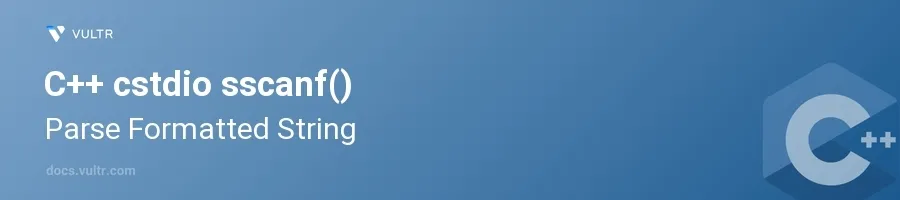
Introduction
The C++ sscanf()
function, part of the <cstdio>
library, is a powerful tool for parsing formatted strings. This function allows you to read data from a string and format it into different variable types based on specified format specifiers. It's highly useful in scenarios where input strings contain structured data, and you need to extract elements and convert them into usable formats programmatically.
In this article, you will learn how to effectively use the sscanf()
function to parse various data types from strings. Explore key usage strategies, from basic to more complex scenarios, enhancing your ability to handle and convert formatted string data efficiently in C++.
Understanding sscanf() Basics
Parse Integer Values
To parse integer values from a string using sscanf()
, follow these steps:
Prepare a char array that contains the string with the integer.
Use
sscanf()
to extract the integer into a variable.cppchar str[] = "Age: 45"; int age; sscanf(str, "Age: %d", &age);
In this code snippet,
%d
serves as a format specifier forsscanf()
to recognize and extract the integer.age
will hold the value45
after parsing.
Extract Floating Point Numbers
To handle floating point values:
Designate the string containing the float.
Apply
sscanf()
to parse the float value correctly.cppchar info[] = "Value: 3.62"; float value; sscanf(info, "Value: %f", &value);
Here, using
%f
tellssscanf()
to parse a floating point number, resulting in3.62
being assigned tovalue
.
Advanced String Parsing Techniques
Combining Multiple Types
For strings with multiple data types:
Set up a string that includes different types of data.
Use
sscanf()
to parse each based on the correct format specifiers.cppchar details[] = "John 32 180.5"; char name[10]; int age; double height; sscanf(details, "%s %d %lf", name, &age, &height);
%s
reads a string until the first whitespace,%d
reads an integer, and%lf
reads a floating type number. John’s name, age, and height are parsed respectively.
Parsing Dates
For more structured data like a date:
Start with a string embedding a date.
Use the correct specifiers to extract year, month, and day.
cppchar date_str[] = "2023-03-15"; int year, month, day; sscanf(date_str, "%d-%d-%d", &year, &month, &day);
The
%d
specifiers allow for parsing integer values from the string formatted as a date.
Conclusion
Utilize the sscanf()
function in C++ to transform structured string data into variously typed variables efficiently. By mastering this function, you optimize data processing involving formatted strings, bolstering both the versatility and reliability of your codebase. Adopt the techniques demonstrated to parse integers, floats, strings, and more complex structured data like dates, ensuring that your applications can handle diverse input formats accurately.
No comments yet.