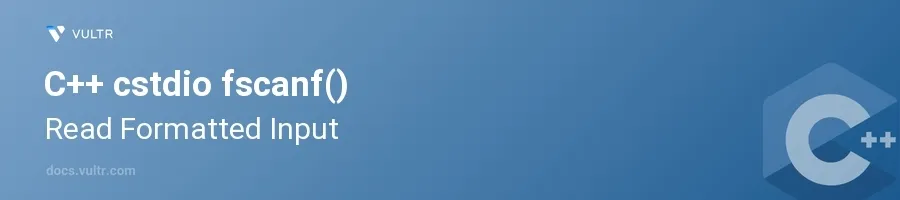
Introduction
The fscanf()
function in C++ is a crucial tool for reading formatted input from a file. It is part of the C standard library, accessible via the <cstdio>
header, and provides a convenient way to read data formatted as in printf()
. This ability to specify the type and format of input data makes fscanf()
highly versatile for parsing files with known structures.
In this article, you will learn how to effectively use the fscanf()
function in C++ to read various data types from a file. Explore practical examples that illustrate reading different kinds of formatted input using fscanf()
, enhancing your ability to handle file input operations in your C++ applications.
Understanding fscanf()
Basic Usage of fscanf()
Include the necessary header and open a file using
fopen()
.Use
fscanf()
to read data from the file, specifying types appropriately.cpp#include <cstdio> FILE *file = fopen("data.txt", "r"); int integer; float floating_point; char str[100]; fscanf(file, "%d %f %s", &integer, &floating_point, str); printf("Read integer: %d, floating point: %f, string: %s\n", integer, floating_point, str); fclose(file);
In this code snippet,
fscanf()
reads an integer, a floating point number, and a string from a file nameddata.txt
. The formats%d
,%f
, and%s
correspond to the types of the respective data being read.
Reading Complex Formatted Data
Prepare to read more structured data, including loops for multiple entries.
Use a while loop to continuously read until EOF is encountered.
cpp#include <cstdio> FILE *file = fopen("complex_data.txt", "r"); int id; double salary; char name[100]; while(fscanf(file, "%d %lf %99s", &id, &salary, name) == 3) { printf("ID: %d, Salary: %.2lf, Name: %s\n", id, salary, name); } fclose(file);
Here, the
while
loop usesfscanf()
to read each entry until it reaches the end of the file. It checks if all three inputs (ID, salary, and name) are successfully read by confirming that three items (3
) are processed.
Handling Errors and File End
Implement error checking in your file reading operations.
Check the result of
fscanf()
to handle file ends and read errors effectively.cpp#include <cstdio> FILE *file = fopen("data.txt", "r"); int data; if (file) { while(fscanf(file, "%d", &data) != EOF) { if (ferror(file)) { perror("Error reading from file"); break; } printf("Data: %d\n", data); } fclose(file); } else { perror("Failed to open file"); }
This example adds error handling for file operations. It checks if the file opened successfully and handles potential read errors by monitoring the return value of
fscanf()
.
Conclusion
Master the fscanf()
function in C++ to enhance your file input capabilities. By understanding and utilizing its format specifiers and handling its operational nuances like file ends and error detection, you optimize data ingestion tasks in your programs. Apply the aforementioned strategies to deal with structured and unstructured data efficiently, ensuring robust and error-free file reading in your applications.
No comments yet.