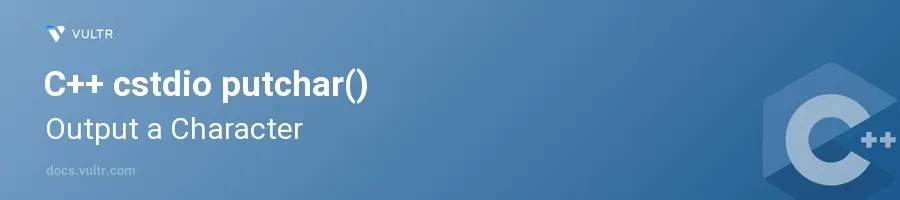
Introduction
The putchar()
function from the C++ <cstdio>
library provides a straightforward way to output a single character to the standard output, typically the screen. This function plays a pivotal role in situations where individual character handling is necessary, particularly in text-based interfaces or simple outputs during debugging.
In this article, you will learn how to use putchar()
in your C++ programs effectively. Explore different scenarios where this function can be applied, including outputs within loops and conditionals, enhancing code readability and efficiency in character processing tasks.
Basic Usage of putchar()
Output a Single Character
Include the
<cstdio>
library in your program.Use the
putchar()
function to output a character.cpp#include <cstdio> int main() { putchar('A'); return 0; }
This code snippet will output the character
A
to your console. Theputchar()
function writes this character to standard output.
Output Multiple Characters in a Loop
Utilize loops to repeatedly call
putchar()
.Plan for each iteration of the loop to output a distinct character.
cpp#include <cstdio> int main() { for (char ch = 'A'; ch <= 'Z'; ch++) { putchar(ch); } return 0; }
In this example,
putchar()
is used within a for-loop to print all the uppercase letters from 'A' to 'Z'. Each character is sent to the standard output one after another, creating a sequence.
Advanced Applications of putchar()
Using putchar() with Conditional Logic
Add conditionals to determine which characters to output.
Integrate
putchar()
within these conditions to control output dynamically.cpp#include <cstdio> int main() { for (char ch = 'A'; ch <= 'Z'; ch++) { if (ch % 2 == 0) { // Check if the character code is even putchar(ch); } } return 0; }
This code will output every second letter from 'A' to 'Z', i.e., 'B', 'D', 'F', etc. The
if
conditional checks whether the ASCII value of the character is even before usingputchar()
to output it.
Combining putchar() with Other Functions
Combine
putchar()
with other C++ IO functions for more complex output formatting.Ensure that
putchar()
is appropriately synchronized with other output functions likeprintf()
orcout
.cpp#include <cstdio> #include <iostream> int main() { std::cout << "Alphabet: "; for (char ch = 'A'; ch <= 'Z'; ch++) { putchar(ch); } std::cout << std::endl; return 0; }
This code uses
std::cout
to print a prefix "Alphabet: " before usingputchar()
to output all letters from 'A' to 'Z'. After the loop, it outputs a newline viastd::cout
.
Conclusion
The putchar()
function in C++ is an essential tool for outputting individual characters to the console. By incorporating this function effectively within loops, conditionals, and in conjunction with other output functions, you optimize your C++ programs for tasks involving direct character manipulation. Whether you're generating simple outputs or engaging in complex character data processing, understanding and using putchar()
enhances your programming capability in C++. Embrace these techniques to ensure your code is not only functional but also intuitive and efficient.
No comments yet.